Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial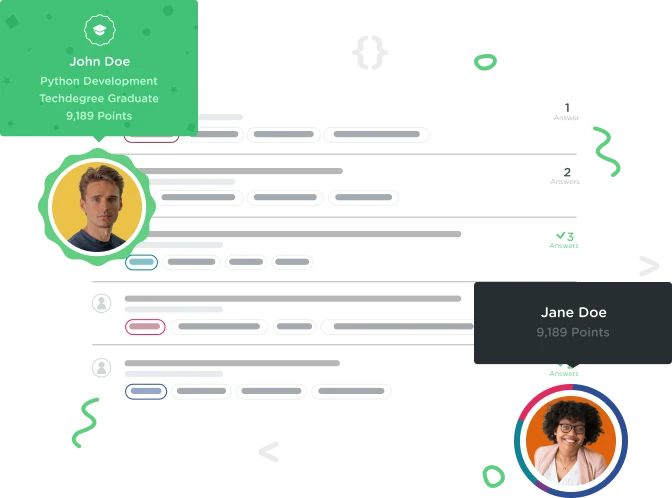

Richard Jones
3,500 PointsStuck on Frog Tongues. I am getting compile errors that don't show up in Visual Studio.
Hi, Can someone give my code a second peek? It says I have compile errors and I am not sure why. Thank you!
using System;
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double GetAverageTongueLength(Frog[] frogs)
{
double sumTongues = 0.0;
double result = 0.0;
for (int i = 0; i < frogs.Length; i++)
{
sumTongues += double.Parse(frogs[i]);
}
result = sumTongues / frogs.Length;
return result;
}
}
}
namespace Treehouse.CodeChallenges
{
public class Frog
{
public int TongueLength { get; }
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
}
}
3 Answers
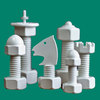
Steven Parker
230,688 PointsYou're pretty close, but...
- you don't need double.Parse because the lengths are already numbers (not strings)
- you can't add the frog itself, you need to select the TongueLength property

Richard Jones
3,500 PointsOh, I thought I understood objects, Now I am not sure how to grab a ToungeLength property out of the object Frogs[].
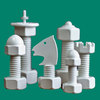
Steven Parker
230,688 PointsThe "dot notation" is a most common way to select a property: "objectname.propertyname
". Or in this case, since you're also selecting an object from an array with an index: "objectarrayname[index].propertyname
".
And don't forget your object array is frogs (with a lower-case "f").

Richard Jones
3,500 PointsThank you, Steven. I changed my sumTongues to an int so I didn't have to convert, I then accessed the toungueLength as you stated. Finally, divided as before and made it a double called result. Worked. I guess when you divide two ints and the answer is a double you can use double.