Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial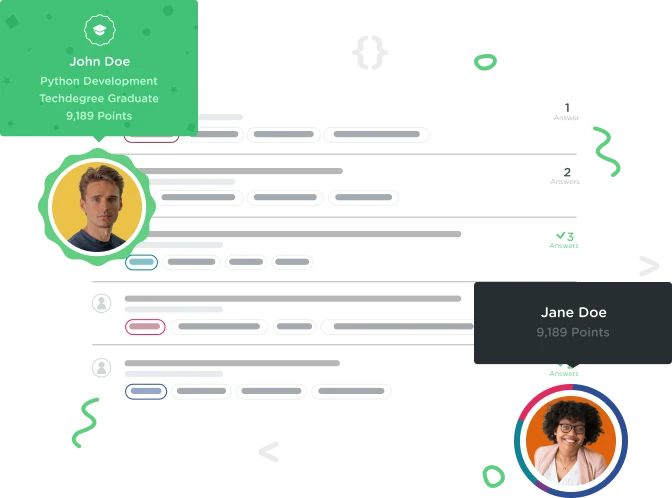
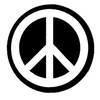
john larson
16,594 PointsStuck on one part of a list.remove() function
Trying to add a function to remove an item from a list. I'm just stuck at how to exit. In my example I'm using numbers and passing in the numbers to remove through an input/int. if I try to exit with say a 0, it get a numbers not in the list error. If I use a word like exit it's not a number so I get that error. Same thing if I pass an empty string. So, wondering what I could use to exit. Miraculously the code seems to be working to this point :D
entry = input(">")
num_list = [1,2,3,4,5]
if entry.lower() == "remove":
while True:
def remove_num(num):
num_list.remove(num)
num = int(input("Enter the item to be deleted, press enter to exit: "))#stuck here
remove_num(num)
if num == "": #don't know what to use to exit
break
print(num_list)
3 Answers
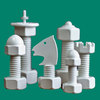
Steven Parker
229,783 Points
You could check for an empty string before you convert to a number:
answer = input("Enter the item to be deleted, press enter to exit: ")
if answer == "":
break
num = int(answer)
remove_num(num)
This replaces lines 7-10 in the original code.
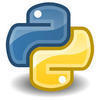
Gerald Wells
12,763 PointsHierarchy and specifying type
If you want to catch a value like 0
to have the loop break, it needs to be the first condition you test. Python is a scripting language that works from the top down. since 0
is an integer which you are requiring on input, it will try and remove it from the list, since 0
is not in the list it will throw an error.
The type of value that needs to be mutated in your collection is only relevant to your function remove_number
's scope. requiring it on input will become a headache, you could always use a try
and except
block to ensure the input is True but at that point your algorithm is becoming increasingly more inefficient. The purpose of functions is to make them portable to other applications, by naming your function remove_number
you know that it will never be used to remove a character value, therefore type int
is only relevant to that function.
Defining functions
It is bad practice to define a function inside your while
loop. Will it work? yes, but if you are exiting it is inefficient to have the program read through the function again, it should only be read when needed.
Hope this helps!
num_list = [1,2,3,4,5]
def remove_num(num):
return num_list.remove(int(num))
def get_remove():
num = input("Value to remove? ")
try:
if num != "" and int(num) in num_list:
remove_num(num)
get_remove()
else: pass
except ValueError:
again = input("Thats not a number...exit?[yN] ").lower()
if again != "y":
get_remove()
if __name__ == '__main__':
entry = input(">").strip().lower()
if entry == "r":
get_remove()
print(num_list)
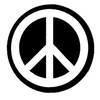
john larson
16,594 PointsGerald, thank you. That's more information than I was looking for at the moment, But a very good and thorough answer .

Alexander Kobilinsky
359 Points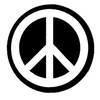
john larson
16,594 PointsHey Alexander, I followed that link..not sure what that's about.
john larson
16,594 Pointsjohn larson
16,594 Pointsactually, I think it does work...but I get a nasty error message. So I'd like to get rid of that. Also I tried using -1 to exit thinking maybe I could bypass that the number wasn't in the list..but that still gave an error "not in list"