Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial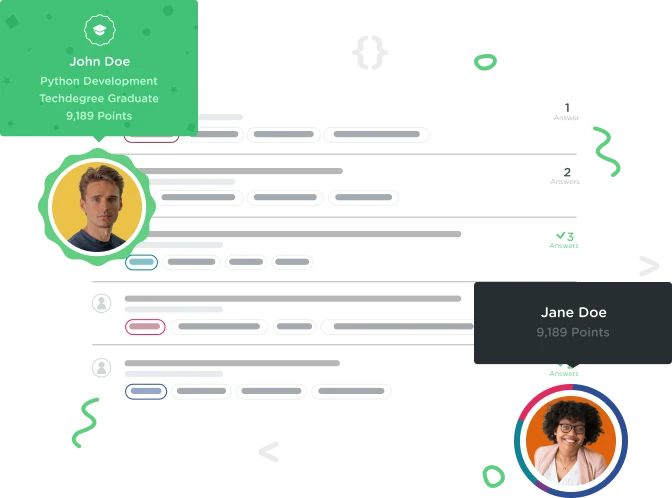

Peter Nguyen
591 PointsStuck on Python Basics Challenge
Not sure how to do this one
Q: Write a function named printer. The function should take a single argument, count, and should print "Hi " as many times as the count argument. Remember, you can multiply a string by an integer.
7 Answers
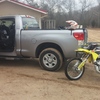
Ryan Ruscett
23,309 PointsHola man,
Let me explain this best I can. Thanks for choosing me to answer your question. Ok let's get to it.
Let's look the question and break it down.
Write a function named printer. The function should take a single argument, count, and should print "Hi " as many times as the count argument. Remember, you can multiply a string by an integer.
Ok, break down.
- Write a function called printer.
- The function takes 1 argument which is counter.
- Print the word "Hi"
- Print the word "Hi" as many times as whatever the counter argument is.
Ok so with that in hand. Let's do it.
- Write a function called printer
def printer():
We know that to declare a function we need to use def keyword signifying its a function with ().
- The function takes 1 argument called counter. Ok cool.
def printer(counter):
An argument goes in the () of the function. Meaning that the function needs a value (whatever value is in the()) in order to run do processing.
- Let's print the world Hi.
def printer(counter):
print("Hi")
Ok, awesome. We now have a function called printer that takes a single argument counter, and it also prints the word "Hi".
- Print the word as many times as the counter says. We don't know what the counter is but we don't really care do we? As the questions says, we can multiply the string by an integer. A quick few points.
Point 1: print("Hi") we know this is a string, because Hi is surrounded with quotes. "Hi", signifying it's a string. But if am to write the string out x amount of times. x would have to be a number right?. Lets print Hi 5 times or 8 times. I mean I can't print Hi (use another string) "apple" times now can I? No of course not how do I do "Hi" "apple" times. I don't.
So I need to print the word Hi as many times as whatever the value for count is. Which brings me back to the questions which says I can multiply a string by an integer. As in my example above, the string is "Hi" and it only makes sense to multiply that by an integer, since we learned that we can't multiply it by another string, that makes no sense.
def printer(count):
print("Hi" * counter)
BAM! We have our answer. We use an Operator called * This operator means multiplications. For example
y = 10 * 10
Y is actually equal to 100. So if I say, hey Peter. I want you to say Hi ten times. You say hi, hi, hi etc. You say it ten times. So the program way to say, say hi ten times is what is Hi times ten AKA (Hi * 10). It's Hi Hi Hi HI Hi Hi HI HI HI Hi.
I hope this helps. I am not sure where your level is in the game of python, so PLEASE feel free to tell me that you have no idea what I am talking about, or that you get it buuuttttt..... I am happy to help in anyway that I can.
Thanks!

Ken Alger
Treehouse TeacherPeter;
Welcome to Treehouse!
Let's take it one step at a time.
First, define a function named printer
that takes one argument, count
. We can do that right?
def printer(count):
Second, we want to print "Hi" as many times as the count
argument. In Python if we do 'Hi ' * 3
we will get Hi Hi Hi
. So to continue our code then...
def printer(count):
print("Hi " * count)
That should do it. Please post back if any of that did not make sense.
Happy coding,
Ken

Scott Halford
8,964 PointsThank you Ken for your helpful response.

Mark Bell
7,052 PointsI had this issue. I have noticed once or twice that something comes up in the quiz/code challenge that we haven't covered in the course. In this case, the very next video covered the all important ' * count' action that you were missing in the initial challenge. Kenneth is pretty good though I have to admit and the odd snag is no bother at all. I had never intended to learn Python but a recent IoT project has forced my hand and is proving to be a very smooth addition to my armoury with KL's help!
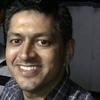
Sandeep Krishnan
9,730 PointsI agree. It happened in many other tracks too.
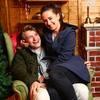
Patric Daniel Pförtner
1,542 PointsHi Peter,
You are very close to the answer, here is how I did it:
def printer(count):
print("Hi " * count)
Why are you learning Python? Maybe we have the same aims! I am learning it to bring my Start UP www.wolf-gate.com to the next level. Thank´s to Kenneth Love it´s easily possible :)

kenyetta watt
6,899 Pointsi tried all of these and none of them were correct

Daniel Walker
2,031 PointsKenyetta Watt, i can help you. just comment and ill be able to help.

Marvin Calderon
710 PointsAm i crazy to assume that "count" is just a place holder for an integer?

sepand h
880 Pointsyou can do it with a loop also.
def printer(n):
while n:
print('hi')
n -= 1
printer(4)
Scott Halford
8,964 PointsScott Halford
8,964 PointsThank you Ryan! for your thorough explanation. This helped me tackle the middle and latter part of this challenge as I didn't know what to do after: def printer (count):
Cristobal Ortiz-Ortiz
1,000 PointsCristobal Ortiz-Ortiz
1,000 Pointsso the default value of count is ? exercises are little confusing :PP
Sandeep Krishnan
9,730 PointsSandeep Krishnan
9,730 PointsHello Ryan Ruscett - Thank you for your detailed explanation. I am clear as crystal.
Bala Selvam
Python Development Techdegree Student 30,590 PointsBala Selvam
Python Development Techdegree Student 30,590 Pointswhy does the def have count and the print have counter in it, shouldnt that be count as well?
Kevin Lieu
4,329 PointsKevin Lieu
4,329 PointsWOW. Methodical and thorough explanation. Thank you.
Trey Robison
Courses Plus Student 481 PointsTrey Robison
Courses Plus Student 481 PointsThank you for the breakdown. I am a beginner and appreciate the support.
Christopher Bailey
14,880 PointsChristopher Bailey
14,880 PointsCan someone explain to me why this is wrong,,,
def printer(count): print("Hi ")*count
But this is correct
def printer(count): print("Hi " * count)
Even though they care the same results??? I tested both on my Local install of Linux and running the latest python installation... both bare the same results.. but only one answer was correct for the quizlett...
But why...
thanks all
Michael Fazio
15,586 PointsMichael Fazio
15,586 PointsBest. Answer. Ever! Thanks!