Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial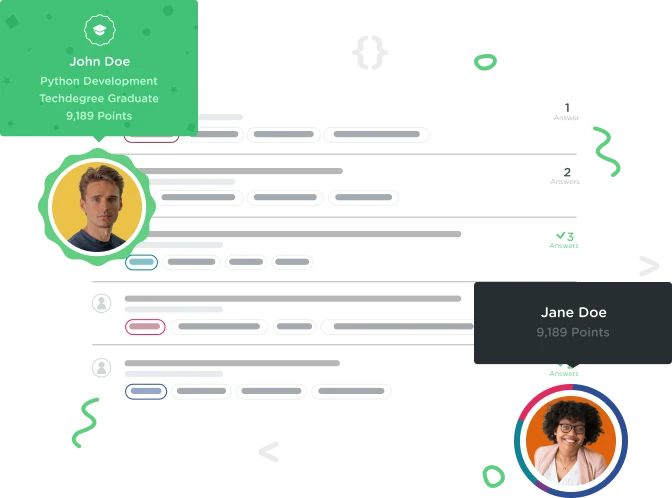
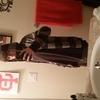
Jt Miller
1,242 PointsStuck on remainders
This is what it asks:
This one should be short and sweet. Write a function named even_odd that takes a single argument, a number. return True if the number is even, or False if the number is odd. You can use % 2 to find out the remainder when dividing a number by 2. Even numbers won't have a remainder....
I start by creating the function like it asks then it says return true or false if it has a remainder so I set number to 7 an odd number so it will then I create an if statement and I am kinda confused on what to do as I know I'm not on the correct path.
def even_odd(number):
number = 7
if number == % 2:
return True
else:
return False
even_odd(number)
1 Answer
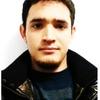
Anibal Marquina
9,523 Points#Your code is almost correct, you need to remove (number=7) from the block...
#if you want to try your code you can pass 7 as the argument print(even_odd(7)) in your local python shell.
# Also, take a look at the syntax of your if statement.
def even_odd(number):
if number % 2 == 0:
return True
return False
Christian A. Castro
30,501 PointsChristian A. Castro
30,501 PointsAnibal Marquina Thank you so much for your advice!! However I still a bit confused with your code above. Could you be able to explain the last two returns? How did you were able to put two returns under the same section? :/ I got all lost on that part!
Anibal Marquina
9,523 PointsAnibal Marquina
9,523 Points