Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial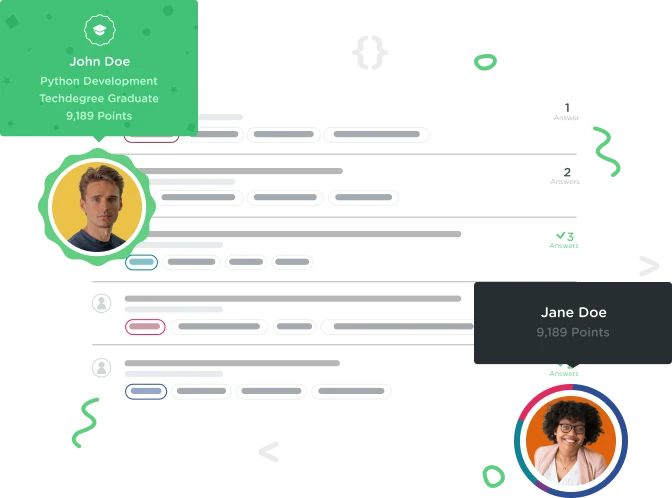

taejooncho
Courses Plus Student 3,923 PointsStuck on RPG roller challenge part 2
Hello,
I am stuck on this challenge. I think I have the codes right, but when I try to check the work I get Bummer! Can't get the length of a 'Hand'
I would like some guidance on where I am wrong. Please do not give me answers for this challenge, I would like to know and really solve this.
I would also like to know if there is any difference between these two codes
def __init__(self, size = 0, die_class = D20):
super().__init__()
for _ in range(size):
self.append(die_class())
and
def __init__(self, size = 0, die_class = None):
super().__init__(size = 20, die_class = D20)
Below this line are the codes that I have written for this challenge.
import random
class Die:
def __init__(self, sides=2):
if sides < 2:
raise ValueError("Can't have fewer than two sides")
self.sides = sides
self.value = random.randint(1, sides)
def __int__(self):
return self.value
def __add__(self, other):
return int(self) + other
def __radd__(self, other):
return self + other
class D20(Die):
def __init__(self,size=20):
super().__init__(size)
from dice import D20
class Hand(list):
def __init__(self, size = 0, die_class = None):
if not die_class:
raise ValueError("You need to have die_class")
super().__init__(size = 20, die_class = D20)
@classmethod
def roll(cls, number):
if number > 0:
return cls(size = 20, die_class = D20)
number -= 1
@property
def total(self):
return sum(self)
1 Answer
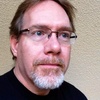
Chris Freeman
Treehouse Moderator 68,423 PointsIn both __init__
examples, super()
refers to the parent class list
. In the second example, super is passing arguments to list
. The class list
does not know what to do with the arguments die_class
or size
so nothing happens with them.
def __init__(self, size = 0, die_class = None):
super().__init__(size = 20, die_class = D20)
So use the first example to initiate the Hand with die_class instances.
You roll
method is SO close. The size argument should be 2 not 20.
Also, the number
decrement is unused and not reachable after the return statement.