Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial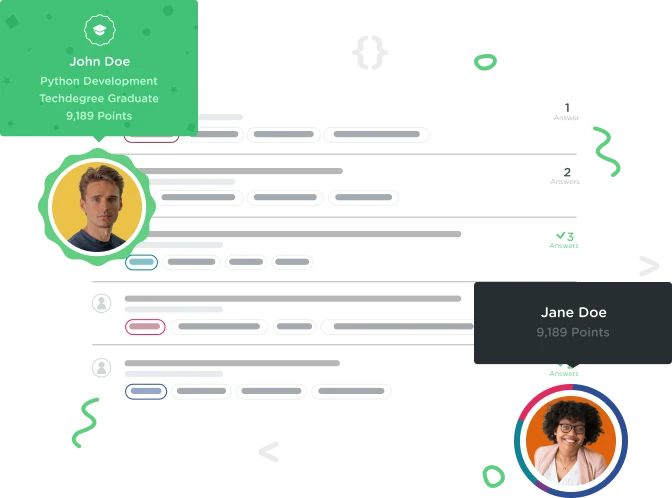
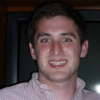
Richard Luick
10,955 PointsStuck on Ruby Core Extra Credit - Movie Class
Hello, I decided to try my luck with the extra credit under the Ruby Core section of the Ruby Foundations track which is as follows:
Now that we have many of the basic classes of Ruby in our toolbox, try writing a program that uses modules and classes to create a movie class. Each movie should have a title and rating. Finally, implement comparison of movies using the Comparable module.
I'm starting out with a simple movie class which takes in the title and rankings of a movie and sends that to a hash. The issue I am having is that when I display the hash, it only displays the second movie and not the first one and the second one. I believe this most likely has to do with some sort of variable scope but I cannot seem to figure it out. Any help is appreciated. Thank you!
class Movies
attr_accessor :title, :rating
def initialize
@@movies = {}
end
def add_movies
@@movies[@title] = @rating
end
def self.display
puts @@movies
end
end
movie1 = Movies.new
movie1.title = "Fargo"
movie1.rating = "10"
movie1.add_movies
movie2 = Movies.new
movie2.title = "Transformers"
movie2.rating = "1"
movie2.add_movies
Movies.display
1 Answer

Jamie McCaw
5,579 PointsThe reason this is happening is because you are basically making 2 movie lists and only displaying the last one.
class Movies
attr_accessor :title, :rating
def initialize
@@movies = {}
end
def add_movies
@@movies[@title] = @rating
end
def self.display
puts @@movies
end
end
movie1 = Movies.new #Creates a new Movie object called movie1
movie1.title = "Fargo" #Adding movie data
movie1.rating = "10" #Adding movie data
movie1.add_movies #Adding movie data
# Movies.display here would show you {"Fargo"=>"10"}
movie2 = Movies.new #Here is the problem, you are creating a second movie object that the new movie will be placed into
movie2.title = "Transformers"
movie2.rating = "1"
movie2.add_movies
Movies.display # This produces only {"Transformers"=>"1"}
------------------------------------------------------------------------------------
#Cleaner version that does what you want it to
movies = Movies.new
movies.title = "Fargo"
movies.rating = "10"
movies.add_movies
movies.title = "Transformers"
movies.rating = "1"
movies.add_movies
Movies.display
Richard Luick
10,955 PointsRichard Luick
10,955 PointsOkay thanks that makes sense.