Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial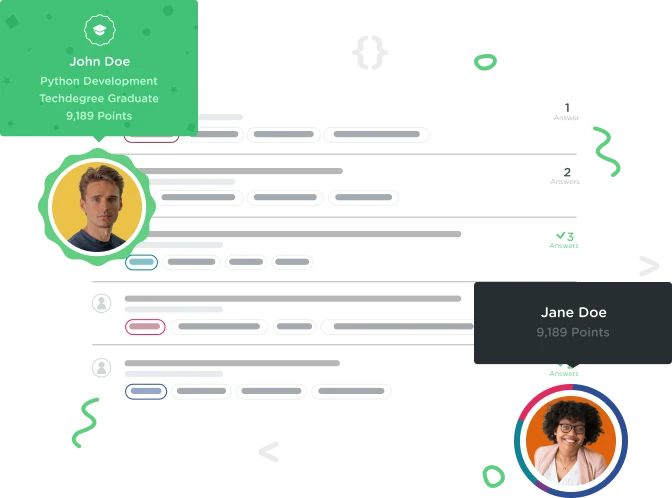

Keenan Smith
Python Development Techdegree Student 5,487 PointsStuck on SortedInventory class
looking in my notes I thought this is what it is asking us to do. I keep getting a bummer error though. What am I doing wrong?
class Inventory:
def __init__(self):
self.slots = []
def add_item(self, item):
self.slots.append(item)
class SortedInventory(Inventory):
def __init__(self, item):
super().add_item(self, item)
self.slots.append(item)
1 Answer
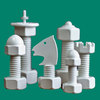
Steven Parker
231,275 PointsThe instructions ask you to "override the add_item method.", but this code is overriding "__init__" instead.
Also, you won't need to call "append" yourself, since it will be done by the "super" call.
Keenan Smith
Python Development Techdegree Student 5,487 PointsKeenan Smith
Python Development Techdegree Student 5,487 PointsOk, so I think now I understand what it was asking for when it said override. Thank you. now after fixing that and eliminating the append code, im getting an error that says: "Bummer: You need to override
add_item
inside ofSortedInventory
. For now, just have it callsuper()
"Is it not overriding even though I'm creating the method add_items?
Steven Parker
231,275 PointsSteven Parker
231,275 PointsYou need to "override the add_item method.", but this code is defining "add_items" (plural) instead.
And when you call a method, you don't need to pass "self" explicitly. The system adds that for you.
Keenan Smith
Python Development Techdegree Student 5,487 PointsKeenan Smith
Python Development Techdegree Student 5,487 PointsThat worked out. had that not been a challenge could we just have sorted the list within the Inventory class? Or is it better to have a new class do it?
Steven Parker
231,275 PointsSteven Parker
231,275 PointsYou could have added sorting to the original class, but maybe there would be a reason to retain the original order of creation (it's not clear from just the code).