Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial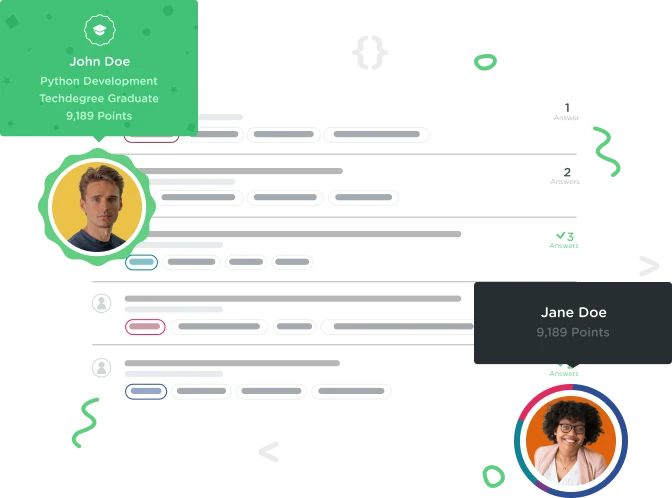
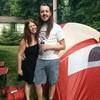
Carter Pringle
3,416 PointsStuck on Stage 4 exercise
The wording is a little weird, for me, so I don't really know what needs to be done?
"So I was wondering if you could help me with some of these code challenges. As you might've seen when a member field name doesn't match the style we've talked about, I send an error about it. Let's write some code to validate the style of the field name.
I've added the method validatedFieldName it will return the validated field name. If the value passed in doesn't meet the requirements, throw an IllegalArgumentException. I'll keep you posted on the results every time you press check work."
3 Answers
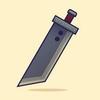
Allan Clark
10,810 PointsThe comments inside the code help a lot: // These things should be verified: // 1. Member fields must start with an 'm' // 2. The second letter in the field name must be uppercased to ensure camel-casing // NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
Basically the method will be passed a String in the argument fieldName. You need to add code to make sure that String meets the 2 above requirements, throw an exception if the String does not meet them.
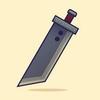
Allan Clark
10,810 Pointsif (fieldName.startsWith("m")){
Field name is a String, it does not come with a startsWith() method. You could create your own private method that would do this but String comes with a way of accessing individual characters with the charAt() method. Using charAt() should save you writing the extra method lines.
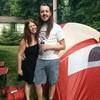
Carter Pringle
3,416 PointsOk, every couple of days I give this thing another shot, and it's frustrating how one little exercise is holding me back. Anway I can get a 'by'?
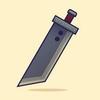
Allan Clark
10,810 Pointsif (fieldName.startsWith("m")){
The starsWith() method isn't on the String API:
http://docs.oracle.com/javase/7/docs/api/java/lang/String.html
To check the first letter is an m you would need to use the charAt() method and logical operators. Remember the it is 0 indexed so the first letter in the String is at 0. Replace the above code with this:
if(fieldName.charAt(0) == 'm') {
This grabs the first letter of the string as a char so you can compare it directly to the 'm' char.
Carter Pringle
3,416 PointsCarter Pringle
3,416 PointsShouldn't this work? It's giving me an error