Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial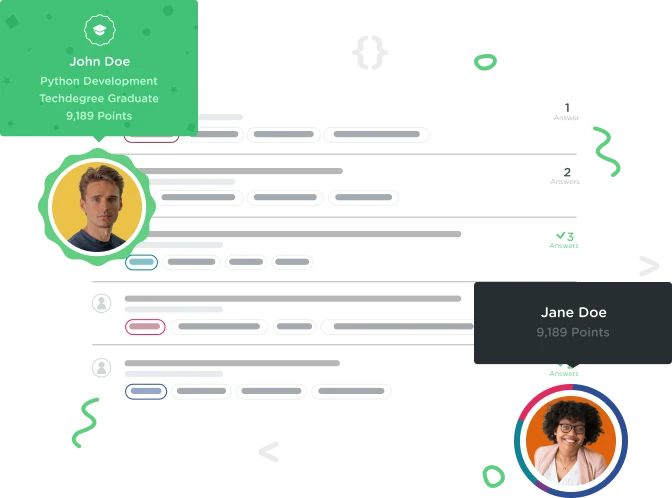
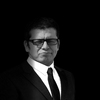
Jose Salazar
Front End Web Development Techdegree Student 13,530 PointsStuck on Stage 5 Functions Quiz...
The exact question is:
Around line 17, create a function named 'arrayCounter' that takes in a parameter which is an array. The function must return the length of an array passed in or 0 if a 'string', 'number' or 'undefined' value is passed in.
Thanks.
9 Answers

Chris Shaw
26,676 PointsHi Jose,
The question in context is simply asking for you to return only the length of an array, if the type of the value is anything other than that of an Array
then return 0
, below is a starting point for how you could structure your answer.
function arrayCounter(value) {
if (typeof value === typeof [] && 'length' in value) {
return value.length;
}
return 0;
}
function arrayCounter(value) {
if (typeof value === 'object' && 'length' in value) {
return value.length;
}
return 0;
}
Both of these answers will work but I'll point your attention to something you may not know yet but you will pick up on your JavaScript journey, if you look at the examples they both check the typeof
of value
. In JavaScript this this perfectly valid but the result you get can be extremely confusing.
For instance lets take the following.
function arrayCounter(value) {
if (typeof value === typeof [] || typeof value === 'object') {
return value.length;
}
return 0;
}
If we were to pass an array to this it would pass both the left and right side of the OR
operator, why? In JavaScript an Array
is a child of the Object
type which can lead to unexpected results since Object
instances have no property called length
which can result in broken code.
To get around this I like using instanceof
which is very easy to use and understand, essentially it allows you to check if the instance of the Object
you have given matches up with a pre-existing Object
type in your code, see the below example.
function arrayCounter(value) {
if (value instanceof Array) {
return value.length;
}
return 0;
}
This is much nicer as it always ensures you're looking for an instance of an Array
and nothing else, we can even cut the code down to 3 lines by doing the following but it's a little harder to understand.
function arrayCounter(value) {
return (value instanceof Array && value.length) || 0;
}
Hope that helps.
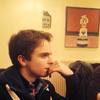
Stephen Ball
4,135 PointsHi Jose,
I was just stuck on this challenge and found it very frustrating. It took some tweaking and changing things, but I eventually got it using this code. Hope it helps!
function arrayCounter(a){
if (typeof a === 'number'){
return 0;
}
if (typeof a === 'string'){
return 0;
}
if (typeof a === 'undefined'){
return 0;
}
return a.length;
}

Chris Shaw
26,676 PointsHi Stephen,
The main issue with the code above is it's very redundant, my post above shows a few different options to complete the challenge but my second last and last examples show a much nice way of writing this function.
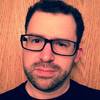
Chris Shaffer
12,030 PointsChris Upjohn I think you should read my response to Dave McFarland from my other thread. Your response saying any response in this thread can be used to pass the challenge AGAIN misses the point.
Getting past the challenge isn't learning. Understanding WHY you got past the challenge in the context of the course - that's learning.
Here's my response from the other thread:
** Your answer still misses the main point: up until this point, although I know the OR operator having done JS courses in places other than Treehouse (I'm just slagging through the Front End Web Dev course for points and to get to the things I haven't learned), the OR operator has NOT actually been taught in the JS Foundations course.
So again, you've provided an answer which does not fit in the context of the lesson.
I think what is missing here is that you guys don't seem to understand that throwing out an answer that says "well here's a way to do it" but doesn't remain in context ANSWERS the question, but leaves us just as confused.
The core of the issue here isn't this particular question but actually two-fold:
1) Jim Hoskins' form of instruction is a mess; he jumps all over, includes ways to NOT do something but still spends 3 or 4 minutes explaining how to NOT do it, and is generally bad at explaining what he is doing and why.... and
2) The JS Foundations course is clearly out of sync with the required material to complete the challenges.
So the question is, do you need to revamp the JS Foundations course questions to match the videos, or are the videos inadequate to provide the skills needed once you reach that point in the course?
Up until this particular track, I've been pretty happy with my experience at TH. At this point, especially after reading of others on this forum with the same frustrations with the same course, and then doing a quick Google search and finding many others who left TH due to what they described as a "mess of a JS course" (posts from over a year ago included), I have to ask if my money might be spent better elsewhere.
If you can't explain the basics of JavaScript and understand the difference between teaching and telling (to be clear: just giving an answer with no explanation - such as your use of the OR operator - is telling) then I'm questioning what my hard-earned money is getting me.
I get it: there's many ways to do things. But if you're teaching a course, then the course should contain the tools and teach the skills needed to pass the required challenges and those things should fit together in a way that results in learning.
I don't feel like I'm LEARNING JS with your method.
**
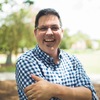
Dave McFarland
Treehouse TeacherI've updated my response below to provide an answer that covers concepts that have all been taught in this course. In fact, @stephen ball's response is spot-on: it solves the challenge and uses basic conditional statements to test the typeof operator:
function arrayCounter(a){
if (typeof a === 'number'){
return 0;
}
if (typeof a === 'string'){
return 0;
}
if (typeof a === 'undefined'){
return 0;
}
return a.length;
}

David Forero
5,492 PointsI am wondering if there is a way to complete the challenge with the code provided in the Javascript Foundations course only. If I am correct that is the main purpose of these challenges.

Chris Shaw
26,676 PointsAll the answers in this thread can be used as the challenge is only looking to ensure the return value is correct, it's not looking for any specific code to get that result.
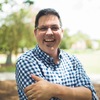
Dave McFarland
Treehouse TeacherTo solve the problem using what you've learned in the course so far, you can use Stephen Ball's answer. To make it less redundant you can combine the various checks for number, string and undefined into a single conditional:
function arrayCounter(a){
var aType = typeof a;
if (aType === 'number' || aType === 'string' || aType === 'undefined'){
return 0;
} else {
return a.length;
}
}
The || symbols are the logical OR operator -- if any of the three conditions are true, the entire condition is true. So this code will return 0 is the passed in argument is a number, string, or undefined.
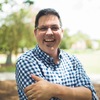
Dave McFarland
Treehouse TeacherAs another student has pointed out -- the ||
(logical OR operator) isn't presented in this course. So Stephen Ball's answer is the best solution for this challenge:
function arrayCounter(a){
if (typeof a === 'number'){
return 0;
}
if (typeof a === 'string'){
return 0;
}
if (typeof a === 'undefined'){
return 0;
}
return a.length;
}
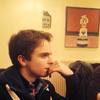
Stephen Ball
4,135 PointsHi Chris,
You're absolutely right. Your code is a lot cleaner, mine is the product of frustration, I was just reading your answer there, and it's very useful. It makes me wonder why, in this particular stage of 'JavaScript Foundations', we aren't introduced to, or taught about instanceof. Hopefully it is something that is covered at a later stage. Thanks for your help!
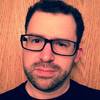
Chris Shaffer
12,030 PointsThis is a perfect example. The challenge immediately after the one in question on this thread requires you cut/paste a function that is already in the code for you.
You LITERALLY copy directly from the video. It's almost the polar opposite of the challenge in this thread, but the result is the same: there's no learning.
Thanks for the replies, guys, but I think I've made my decision. I'll be moving my monthly payment to Code School.
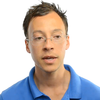
Michael Thomas
10,910 PointsHi
I think when you're a beginner and you're looking for some guidance it doesn't really help when you start throwing things like instanceOf and ||, && that just left me feeling more confused and asking the question, "Oh, did I miss something, when did we learn instanceOf and &&?"
Perhaps, give the answer within the context of the course and then if you like you can say, "hey, here is a more advanced way of doing it, blah blah blah."
Come on guys this is a beginner course, I mean, I'm basically in nappies here hahah! Handle me gently :)
Best wishes, Mike

Blaine Fallis
2,449 PointsI think a challenging question that causes one to research or explore new concepts is what programming is about. I can't imagine there's any programmer that doesn't sometimes or often use resources to solve problems, whether this forum or w3schools or books. This question is a tough one in Foundations stage 5 state of development but led me here to discover some new economical ways of expressing the same thing, new operators (logical "or"), so it's all positive. Thank you EVERYONE in this thread. 95% of the challenge questions are easy so far, but sometimes tough like this one. Maybe that's why it's called a challenge?
Thankful for the helpful participation of Chris U, Chris S, Stephen, Dave, Jose et al. Not to mention Jim Hoskins whose teaching rocks! I'd like to see him create an array called hat and run the pop method to remove a rabbit out of it. Assuming it's the last element in the array of course. Otherwise one might remove a dog out of his hat.
Jose Salazar
Front End Web Development Techdegree Student 13,530 PointsJose Salazar
Front End Web Development Techdegree Student 13,530 PointsMore than helped.
I'm in the same camp as Stephen below in that I was not familiar with 'instanceof.' But, one of the things I have really liked about Treehouse has been the community for being so helpful.
Thanks!
Dominic Bryan
14,452 PointsDominic Bryan
14,452 PointsChris your answer is helpful, but you are jumping the gun on the lessons. I was stuck on this section because the way treehouse represents in the videos and describes it doesn't match the challenge at the end (hence why I ampere looking for the answer). Like I said you are right but at this stage we rant using objects, instance of, &&. to a new beginner if you follow the foundations we haven't seen this before so wouldn't know what they are.
Chris Shaw
26,676 PointsChris Shaw
26,676 PointsI understand where you're coming from Dominic but at the same time these are very basic and easy to understand techniques in JavaScript, the last example as I said is harder to understand but it shows how you can pass the challenge by using popular techniques rather than creating highly redundant code.
Personally I learned all of this by experimenting with it and it made sense very quickly so it's how I perceive the course should have been taught.
Dominic Bryan
14,452 PointsDominic Bryan
14,452 PointsYou are right Christ it is a easy concept, and thanks for telling us because it is helpful. I was just following "by the book" so to speak.