Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial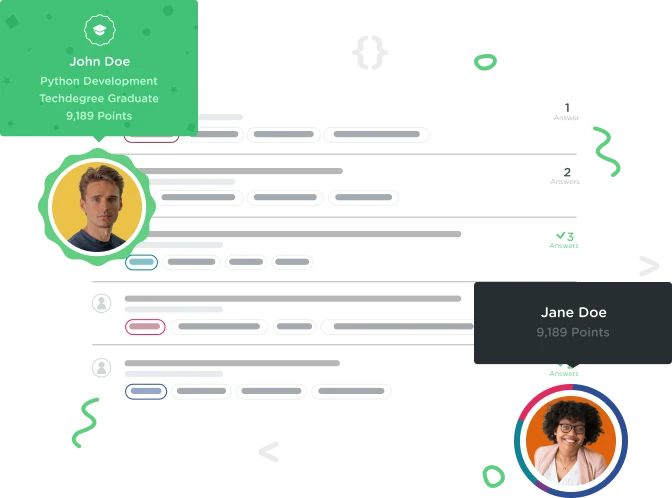

Oğulcan Girginc
24,848 PointsStuck on the 6th OOP Basics Challenge!
Hi,
Once again, I can't figure out what I am missing! Challenge Task 6 of 7 asks me to:
Add a getInfo method to your Trout class that returns a string containing the common_name, flavor, record_weight, and species properties. For example, on our brook trout it might return "Brook Trout tastes Delicious. The record Brook Trout weighed 14 pounds 8 ounces."
Bummer! Try again!
<?php
class Fish
{
public $common_name;
public $flavor;
public $record_weight;
function __construct($name, $flavor, $record)
{
$this->common_name = $name;
$this->flavor = $flavor;
$this->record_weight = $record;
}
public function getInfo()
{
$output = "The {$this->common_name} is an awesome fish. ";
$output .= "It is very {$this->flavor} when eaten. ";
$output .= "Currently the world record {$this->common_name} weighed {$this->record_weight}.";
return $output;
}
}
class Trout extends Fish
{
public $species;
function __construct($name, $flavor, $record, $species)
{
parent::__construct($name, $flavor, $record);
$this->species = $species;
}
public function getInfo()
{
return $this->species . " " . $this->name . "tastes " . $this->flavor . "." . "The record " . $this->species . " " . $this->name . "weighed " . $this->weight . ".";
}
}
$brook_trout = new Trout("Trout", "Delicious", "14 pounds 8 ounces", "Brook")
echo $trout->getInfo();
?>
What am I doing wrong? Can someone please help?
2 Answers
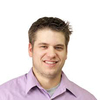
Kevin Korte
28,149 PointsSo two things.
**I did not check you concatenation real well. Assuming that's okay.
Remember that
$name
is part of the __construct function, but in the Trout class $name is set to $common_name. So in yourgetInfo()
you can't ask for it's $name, you have to get it's $common_name. Does that make sense?Earlier you correctly created a variable name
$brook_trout
and make it a new Trout class. The question specifically asks for information on the Brook Trout, where we added as species, so you would run yourgetInfo()
on the$brook_trout
variable, not the$trout
variable of the class.

LaVaughn Haynes
12,397 PointsYour concat is good. You just have a couple of small errors
<?php
//you are missing semicolon at end of next line
$brook_trout = new Trout("Trout", "Delicious", "14 pounds 8 ounces", "Brook")
//as Kevin was suggesting above you want to use $brook_trout below
echo $trout->getInfo();
?>

Oğulcan Girginc
24,848 PointsThank you! :)
Oğulcan Girginc
24,848 PointsOğulcan Girginc
24,848 PointsThank you very much for explanations Kevin!
Kevin Korte
28,149 PointsKevin Korte
28,149 PointsYou're welcome. Funny part is I did this lesson myself just a hour or two before you posted questions on the forum, so this stuff was super fresh in my head.