Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial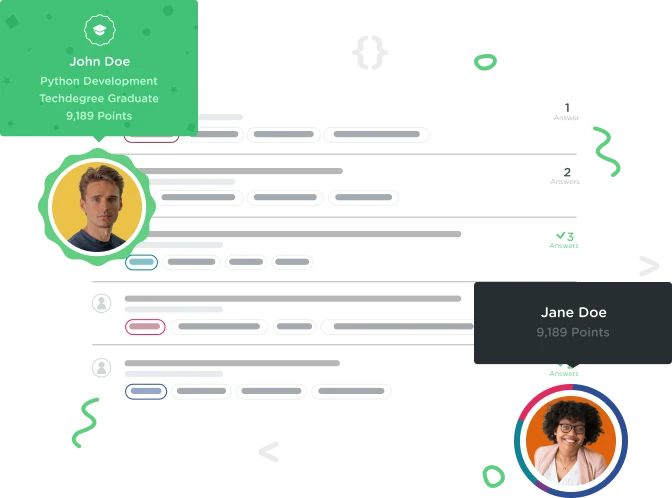

Kap Ok
125 Pointsstuck on the challenge
Don't know what to put in the if statement
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive() {
drive(1);
if () {
throw new IllegalArgumentException("Not enough battery remains");
}
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
1 Answer
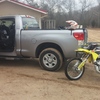
Ryan Ruscett
23,309 PointsHey,
The first issue is you are throwing the error in the wrong method. I am throwing an error if I don't have enough battery to complete the number of laps asked. This means I need to know the number of laps being asked. Since the method is void it doesn't return anything, and it also doesn't take anything. it doesn't take arguments, so there isn't a way for that method to know how many laps you are suppose to do, thus it can't know if it has enough battery.
If you look at the other drive method, that takes arguments. In fact it takes the number of laps as an argument. This is where I want to throw my exception.
If (mBarsCount < laps) -- This says that if the amount of bars in your battery is less than the number of laps, there is no way I can run all the laps being asked. So in that if statement I throw the IllegalArgumentException. In the event that Say I have 4 bars of battery and I need to do 3 laps Than mBarsCount is not less than laps and I can subtract the number of laps from my battery and everything is good.
public void drive(int laps) {
// Other driving code omitted for clarity purposes
if (mBarsCount < laps) {
throw new IllegalArgumentException("Not enough battery remains");
}
mBarsCount -= laps;
}
Does this make sense? If not, let me know!