Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial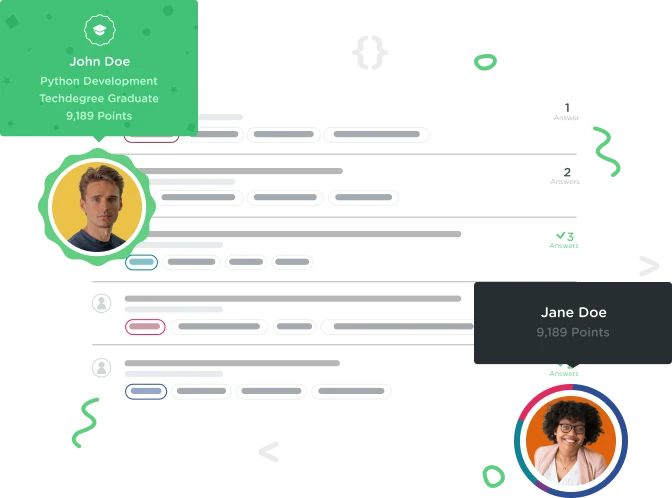

Parker Barandon
377 PointsStuck on this challenge in Swift 3 basics
I'm confused as to why I keep getting this wrong... it is saying that I need to assign the result and not just a bool value. However, anything I try seems to come out as incorrect with the same result. Task 2 is the issue here, Task 1 is apparently fine.
// Enter your code below
let value = 200
let divisor = 5
let someOperation = 20 + 400 % 10 / 2 - 15
let anotherOperation = 52 * 27 % 200 / 2 + 5
// Task 1 - Enter your code below
let result = 200 % 5
// Task 2 - Enter your code below
0 == 0
let isPerfectMultiple = true
20 + 400 % 10 / 2 - 15 ≥ 52 * 27 % 200 / 2 + 5
let isGreater: Bool = false
1 Answer

Jack Baer
5,049 PointsYou are not doing what the tasks are asking. You need to call upon variables in your operations. You just typed
let result = 200 % 5, when what you need to do is type
let result = value % divisor.
Then, you aren't the correct value to isPerfectMultiple. You just did
0 == 0 let isPerfectMultiple = true
0 == 0
literally does nothing
let isPerfectMultiple = true
only assigns true to it, once again not using variable names.
Then you just wrote out the operations and compared them, which is once again a useless statement. And once again, you set isGreater to true, not using variable names.
What you need to have is:
// Enter your code below let value = 200 let divisor = 5
let someOperation = 20 + 400 % 10 / 2 - 15 let anotherOperation = 52 * 27 % 200 / 2 + 5
// Task 1 - Enter your code below let result = value % divisor let isPerfectMultiple = result == 0
// Task 2 - Enter your code below let isGreater = someOperation >= anotherOperation
Your problem is that you need to call upon your variables. Imagine a variable as a box. You put a value in, put a label on that box, and can access its contents using the label name. The name in declaration, such as result, is the label. The contents are the values put inside, so 200 and 5 in the value and divisor variables.
Notice how in my result, isPerfectMultiple, and isGreater declarations, the assigned value is using operators and variable names, not values like 200 or 5.
let result = value % divisor
is the exact same as
let result = 200 % 5
except if you changed the contents of value or divisor later on, the code would fail in the bottom until you manually changed it.
Hope this helps. ~Jack
Josue Gisber
Courses Plus Student 9,332 PointsJosue Gisber
Courses Plus Student 9,332 PointsOk, firstable, the first task is ok. Secondable, on the second task you are not following what the instructions says which is to compare the value of results to 0 and assign that value the constant isPerfectMultiple [
let isPerfectMultiple = result == 0
]