Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial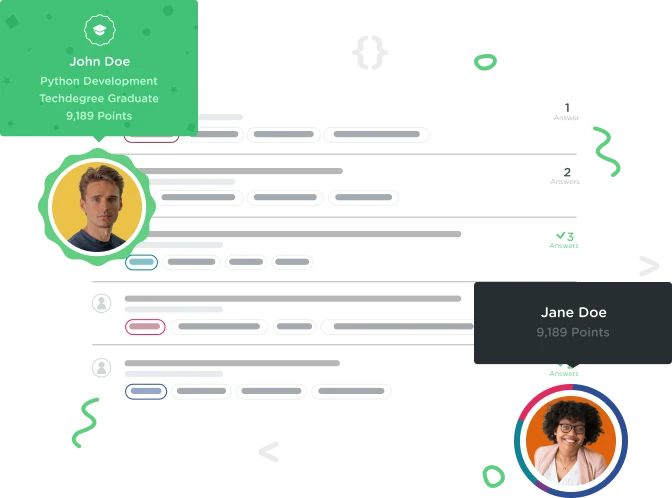

Alex Diaz
4,930 PointsStuck on this challenge, please help!
I've never really been good at stuff like this, please help! I'm very confused as to what i'm even going wrong, thank you.
# Example:
# values = [{"name": "Michelangelo", "food": "PIZZA"}, {"name": "Garfield", "food": "lasagna"}]
# string_factory(values)
# ["Hi, I'm Michelangelo and I love to eat PIZZA!", "Hi, I'm Garfield and I love to eat lasagna!"]
template = "Hi, I'm {name} and I love to eat {food}!"
def string_factory(dicts, **kwargs):
return template.format(name, food)
string_factory(**dicts)
1 Answer

Ryan S
27,276 PointsHi Alex,
The first thing to note is that your function should only take one argument -- a list. Each item in this list will actually be a dictionary.
You will need to loop through the list of dictionaries.
In each iteration of the loop, you will need to unpack that particular dictionary into the .format()
method which will be attached to the template variable. You will need to append this formatted "template" to new list that you will eventually return when the looping is complete.
So you have the right idea with the use of "**
" however, that will be used in .format()
, not as a function argument.
Also, you don't have to call the function at the end. The code checker will do that for you.
Hopefully this clears up the challenge instructions. If it is still unclear then I'd be happy to help you some more.
Alex Diaz
4,930 PointsAlex Diaz
4,930 PointsI'm right here currently, how would I append the results from that for loop to a list? Also where would I use the ** in the format()?
Ryan S
27,276 PointsRyan S
27,276 PointsHey Alex,
You almost got it.
To append an item to a list, use
.append()
on the list.Regarding the "
**
" usage, keep in mind that the variable that you have used to represent each dictionary in the list is "word". So you will need to unpack that variable inside the.format()
method.You can format the string and append it to
new_list
in one line.