Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial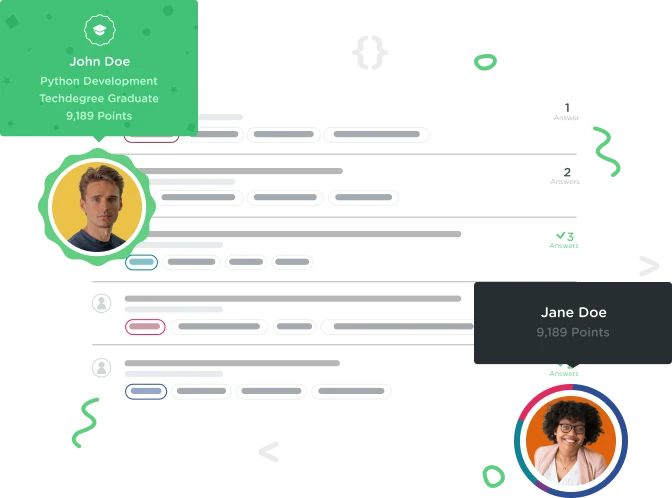
1 Answer
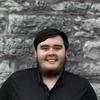
Michael Hulet
47,913 PointsFYI: Next time when you're trying to post code, be sure to wrap it with 3 backticks ( The ` character. It's on the same key as the ~ on most keyboards), and write the language your code is in right after the first 3 backticks, without any spaces. I fixed it for you now, but you can refer to the Markdown Cheatsheet (right under the box where you type a reply) if you have any questions.
Anyway, I see 2 things wrong with your code, both are syntax errors. The first is with your import statement. You wrote it simply as import "Quote.h"
, but the proper syntax is to put an octothorp right before the word "import
", so it looks like this:
#import "Quote.h"
The second thing I see wrong is that you didn't put any character to indicate whether your 2 methods are class or instance methods. This is declared by putting a +
or a -
at the very beginning of the definition. A -
indicates that the method is an instance method, which means that it can only be called on an instance of your class that has already been initialized. This is the most common kind of method. A class method is indicated with a +
, and can be called without an initialized instance of your class having already been created. These are really common when you're writing convenience initializers. If memory serves, both of these should be instance methods, so they should both look like this (with a little unnecessary prettification to my personal preferences):
-(NSArray *) quotes {
if (_quotes == nil) {
_quotes = [[NSArray alloc] initWithObjects:@"1",@"2",@"3", nil];
} return _quotes;
}
-(NSString *) randomQuote {
return @"Some Quote";
}
The entirety of the fixed code should look like this:
#import "Quote.h"
@implementation Quote
-(NSArray *) quotes {
if (_quotes == nil) {
_quotes = [[NSArray alloc] initWithObjects:@"1",@"2",@"3", nil];
} return _quotes;
}
-(NSString *) randomQuote {
return @"Some Quote";
}
@end
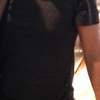
Michael Sevy
3,167 PointsThanks!
Michael Sevy
3,167 PointsMichael Sevy
3,167 Points