Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial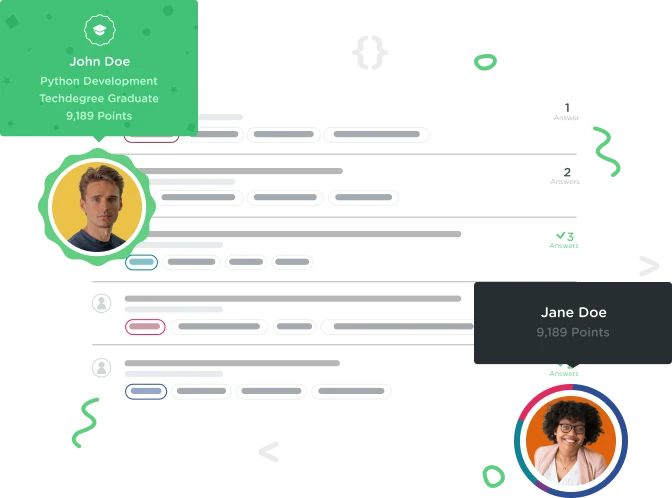

deelan pather
299 PointsStuck on this code challenge Try and Except Challenge 3 of 3 of Python Basics
def add(input1,input2): sum2 = input1 + input2
try: input1 = float(input("Enter a numbers")) except ValueError: print("Those are not numbers")
else: input2 =float(input("Enter a second number")) add() print(sum2)
1 Answer
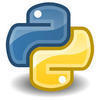
Gerald Wells
12,763 PointsI went and searched for your challenge , next time please post it, also when you post code please format it appropriately. Below I reformatted it but the code really doesn't make sense... I broke down the code line by line and explained what it is that needs to be corrected
Your code:
def add(input1,input2):
sum2 = input1 + input2
try:
input1 = float(input("Enter a numbers"))
except ValueError:
print("Those are not numbers")
else:
input2 =float(input("Enter a second number")) add()
print(sum2)
def add(input1,input2):
This line is good, the only thing I would change if you were trying to develop a project or develop an API, I would be more explicit in my variable naming (Ex: Int_1, int_2). Now if someone is reading this function before they even get into the body of the function they know that it is a function that adds 2 integers. There is a Book called "CleanCode" that I highly advise you read, there will be a link to it at the bottom of this answer.
sum2 = input1 + input2
This line is great, if it was what was asked of you, here you are assuming that both the values you receive are integers. Never assume! The purpose of challenge 3 is attempting to teach you that.
Try and except block....???
try:
input1 = float(input("Enter a numbers"))
except ValueError:
print("Those are not numbers")
May I ask why you are asking for user input? The function received the values that were required, you are negating the purpose of this function when you do this. Another issue with this block specifically on input1
still is if this was the objective you would have to take that input and update a list which is redundant because
A: you already where given the numbers in when the function was called.
B: see A....
Next, except ValueError:
this is correct, however the line that follows is not. The instructions asked you to return None
. You are printing a message to the screen.
...
else block:
else:
input2 =float(input("Enter a second number")) add()
print(sum2)
At this point I think it would be beneficial for you to go back and watch all the python videos again, I know what you are trying to do, but you aren't comprehending the structure of python.
Corrected
Below I have 2 different ways you can do this.
def add(int_1,int_2):
try:
int_1,int_2 = float(int_1), float(int_2)
except ValueError:
return None
else:
return int_1+int_2
######################################
######################################
######################################
def add2(int_1,int_2):
try:
return sum(map(float,[int_1,int_2]))
except ValueError:
return None
Recomended Reading:
- Clean code: https://ebooks-it.org/search-engine.htm?bform=btitle&page=1&query=Clean+Code
- Structure and interpretation of computer programs: http://folk.uio.no/bjornove/sicp.pdf
- Fundamentals of Python: First Programs, Kenneth A. Lambert
- Fundamentals of Python: Data Structures, Kenneth A. Lambert
Gerald Wells
12,763 PointsGerald Wells
12,763 PointsPost a link to the challenge