Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial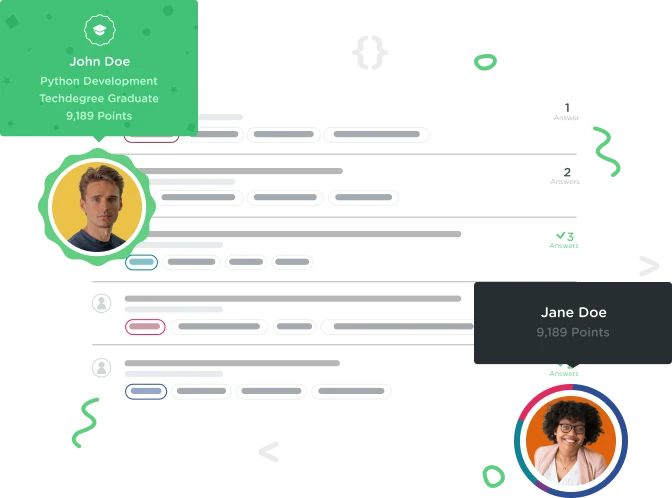

Kade Carlson
5,928 PointsStuck on this one
Is my logic even correct? Not sure what to do here
# combo([1, 2, 3], 'abc')
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
def combo('123', 'abc') {
tuple_list = []
for index in '123' and 'abc':
tuple_list.append('123'[index], 'abc'[index])
return tuple_list
}
4 Answers
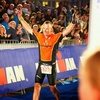
Steve Hunter
57,712 PointsHi Kade,
I approached this one in a similar way to your code.
I started by passing in two iterable parameters, rather than hard-coding the parameters but I doubt the challenge minds but the hard-coded method does make it fiddly to access each iterable.
Inside the method, I initialised a blank list, I called it output
but that's open for renaming!
Next, because I wanted to access the iterables using their index, as you did, I set up a for
loop with a true counter variable that's a number. There are issues with the for in
approach which I'll briefly discuss. Take the other suggested solution here:
for item in first_seq:
# do something with index & square brackets
That won't work. At each iteration, the value held at each element is contained, in turn, within item
. So, you get 1
then 2
etc - these aren't counters; they're the values in the iterable. They could be anything depending on what's passed in. It doesn't use an index approach to accessing the iterable; for starters, the first iterable doesn't start with zero so accessing using []
would fail. The method is supposed to take any iterable, not just [1, 2, 3]
so the method must be able to cope with that. To adopt the approach you've decided on, you need to have the counter variable counting and then access each iterable using [counter]
or [i]
, or whatever you choose.
To do this you need to make the for
loop run on numbers. Because the iterables are both of the same length we can use that to our advantage. Loop through from zero to the length of the iterable and the loop variable (let's call it i
) will count and not hold the values in the iterable.
That could look like:
for i in range(0, len(iter1)):
The variable iter1
is one of the parameters passed in to the method like this:
def combo(iter1, iter2):
So iter1
and iter2
are the same length and can contain the example contents suggested in the challenge, or anything else we choose.
Using the for
loop in this way loops through a range
object which is a range of numbers between the two parameters passed in. The first parameter is zero (the starting index number of the iterable) and the second is the length of iter1
. This will generate a sequence of counter values allowing you to use square brackets to access each element of both, indeed any, iterables.
At the each loop, access iter1[i]
and iter2[i]
to access the relevant parts of each parameter. turn them into a tuple and add that to the blank list you started the method with. I called it output
, remember? To make the tuple, surround the above two iterable accessors with parentheses and separate them with a comma. Also, remember that items in a list are also separated by commas, and that's what we're returning; a list. So, include a comma after the closing parenthesis. Just add that to the output
list with +=
.
Once the loop has finished, return output
.
That all looks like this - which is my solution and by no means the only way to do this:
def combo(iter1, iter2):
output = []
for i in range(0, len(iter1)):
output += (iter1[i], iter2[i]),
return output
I hope that works for you and you understand my ramblings!
Steve.

ds1
7,627 PointsHi, Kade
I'd suggest getting rid of the curly brackets. Also, I see what you're trying to do with the for statement by using 'and' but unfortunately that won't work. The good news is that both sequences will be the same length, which makes it easier... you only have to cycle through one of them in a loop because they're not different lengths. Think about a construct like this to access both sequences to construct your tuples-inside-a-list:
for item in first_seq:
mylist.append((first_seq[i], second_seq[i]))
where 'i' is a counter variable (or you can get a little fancier and use the enumerate function).
[MOD: changed to answer and edited code block - srh]

ds1
7,627 PointsHi, Steve FYI, I think the solution I proposed works also- you just have to add the counter variable 'i' that I was talking about (like below). However, I like your solution better. (When I was working through it myself, I ended up using enumerate() instead of range().) Thanks, D.
def combo(first_seq, second_seq):
mylist = []
i = 0
for item in first_seq:
mylist.append((first_seq[i], second_seq[i]))
i += 1
return mylist
[MOD: added language to code block - srh]
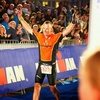
Steve Hunter
57,712 PointsYeah - that works but using a for
loop like that is like having a dog and barking yourself! It can do the counting for you. Using enumerate
is a good option too, yes.

ds1
7,627 PointsBut you're right, using "for item in first_seq" is not ideal. Better to use range() like you did or enumerate(iter1)