Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial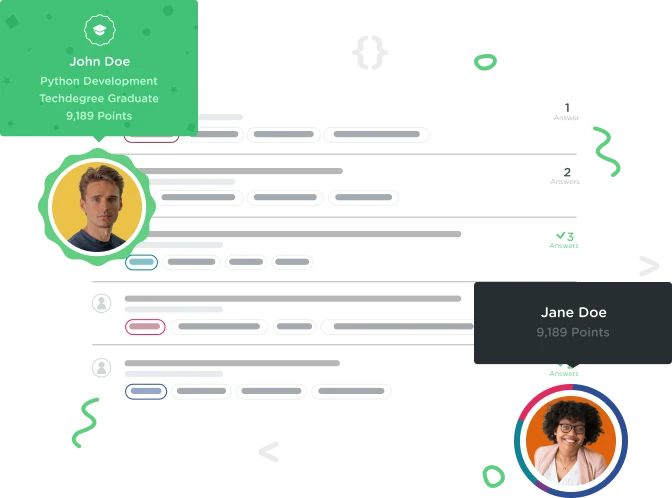

Julius Mickevicius
6,027 PointsStuck on this part with Json. Can anyone point out what i wrote wrong?
Keeps asking if i instantiated a new JsonReader. I believe i'm missing something very obvious here.
using System;
using System.IO;
using System.Collections.Generic;
using Newtonsoft.Json;
namespace Treehouse.CodeChallenges
{
public class Program
{
public static void Main(string[] arg)
{
}
public static List<WeatherForecast> DeserializeWeather(string fileName)
{
var weatherForecasts = new List<WeatherForecast>();
using(var reader = new StreamReader(fileName))
using(var jsonReader = new JsonTextReader(reader))
using(var serializer = new JsonSerializer(jsonReader))
{
weatherForecasts = serializer.Deserialize<List<WeatherForecast>>(jsonReader);
}
return weatherForecasts;
}
public static WeatherForecast ParseWeatherForecast(string[] values)
{
var weatherForecast = new WeatherForecast();
weatherForecast.WeatherStationId = values[0];
DateTime timeOfDay;
if (DateTime.TryParse(values[1], out timeOfDay))
{
weatherForecast.TimeOfDay = timeOfDay;
}
Condition condition;
if (Enum.TryParse(values[2], out condition))
{
weatherForecast.Condition = condition;
}
int temperature;
if (int.TryParse(values[3], out temperature))
{
weatherForecast.Temperature = temperature;
}
double precipitation;
if (double.TryParse(values[4], out precipitation))
{
weatherForecast.PrecipitationChance = precipitation;
}
if (double.TryParse(values[5], out precipitation))
{
weatherForecast.PrecipitationAmount = precipitation;
}
return weatherForecast;
}
}
}
using System;
/* Sample JSON
[
{
"weather_station_id": "HGKL8Q",
"time_of_day": "06/11/2016 0:00",
"condition": "Rain",
"temperature": 53,
"precipitation_chance": 0.3,
"precipitation_amount": 0.03
},
{
"weather_station_id": "HGKL8Q",
"time_of_day": "06/11/2016 6:00",
"condition": "Cloudy",
"temperature": 56,
"precipitation_chance": 0.08,
"precipitation_amount": 0.01
},
{
"weather_station_id": "HGKL8Q",
"time_of_day": "06/11/2016 12:00",
"condition": "PartlyCloudy",
"temperature": 70,
"precipitation_chance": 0,
"precipitation_amount": 0
},
{
"weather_station_id": "HGKL8Q",
"time_of_day": "06/11/2016 18:00",
"condition": "Sunny",
"temperature": 76,
"precipitation_chance": 0,
"precipitation_amount": 0
},
{
"weather_station_id": "HGKL8Q",
"time_of_day": "06/11/2016 19:00",
"condition": "Clear",
"temperature": 74,
"precipitation_chance": 0,
"precipitation_amount": 0
}
]
*/
namespace Treehouse.CodeChallenges
{
public class WeatherForecast
{
public string WeatherStationId { get; set; }
public DateTime TimeOfDay { get; set; }
public Condition Condition { get; set; }
public int Temperature { get; set; }
public double PrecipitationChance { get; set; }
public double PrecipitationAmount { get; set; }
}
public enum Condition
{
Rain,
Cloudy,
PartlyCloudy,
PartlySunny,
Sunny,
Clear
}
}
1 Answer
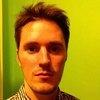
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsI ran your code through as is from the beginning of the challenge, and I got two different complaints from the compiler about this line:
using(var serializer = new JsonSerializer(jsonReader))
1) this serializer isn't IDisposable, so it can't go in the using statement. We should put it inside the block, not outside as another using statement like the others.
2) this constructor doesn't take an argument, we pass in the jsonReader instance later as an argument to the Deserialize method.
using(var reader = new StreamReader(fileName))
using(var jsonReader = new JsonTextReader(reader))
{
var serializer = new JsonSerializer();
weatherForecasts = serializer.Deserialize<List<WeatherForecast>>(jsonReader);
}