Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial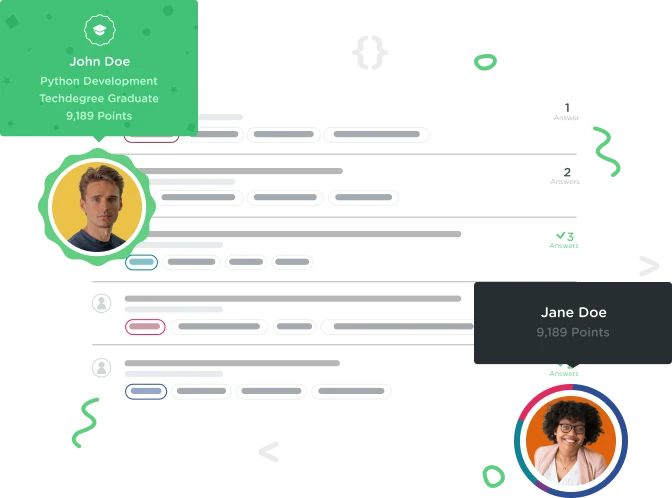
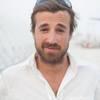
Geert Van Campenhout
7,308 PointsStuck on this Swift Closures task with my higher order function...
I've created the constant "difference" and tried to input the differenceBetweenNumbers function as the parameter for the mathOperation function which does not seem to solve the task. It feels like I'm forgetting something but I'm not sure what it is..
Any help is much appreciated :-(
/**
For this code challenge, letβs define a math operation as a function that
carries out some work on two integers and returns an integer as well. An
example is the function below, `differenceBetweenNumbers`, which takes two
integers and calculates the difference between the numbers. After calculating,
it returns the difference.
*/
func differenceBetweenNumbers(a: Int, b:Int) -> (Int) {
return a - b
}
// Enter your code below
func mathOperation(mathOp: (Int, Int) -> Int, _ a: Int, _ b:Int) -> (Int) {
return mathOp(a, b)
}
let difference = mathOperation(differenceBetweenNumbers)
3 Answers
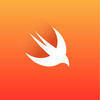
Steven Deutsch
21,046 PointsHey Geert Van Campenhout,
You're close! You just need to pass in the last two arguments for the call your math operation function. It's first argument is a function, which you have successfully passed in differenceBetweenNumbers, now you need to pass in an Int argument for a, and an Int argument for b as well.
func differenceBetweenNumbers(a: Int, b:Int) -> (Int) {
return a - b
}
// Enter your code below
func mathOperation(mathOp: (Int, Int) -> (Int), _ a: Int, _ b: Int) -> Int {
return mathOp(a, b)
}
// We can pass in any two integer values that we want to evaluate
let difference = mathOperation(differenceBetweenNumbers, 20, 10 )
Hope this helps! Good Luck
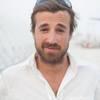
Geert Van Campenhout
7,308 PointsI'm not always sure how the syntax needs to be.. I assumed I had to add the integers themselves but I had tried it like this:
let difference = mathOperation(differenceBetweenNumbers(20, 10))
Cause the parameters of a function are usually between brackets, this made more sense to me.
I guess writing the correct syntax will come over time :-)?
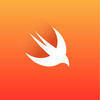
Steven Deutsch
21,046 PointsRight, so let me try to explain better. Your mathOperation has 3 parameters, the first is a function, the second is an Int, and the third is an Int. So when you call this function, you need to pass in 3 arguments separated by commas. You pass it a function as the first argument, a math operation, the specific one being differenceBetweenNumbers. Then you pass in two Integer arguments to be evaluated based on the first math operation argument you passed in. You don't need to pass any values into differenceBetweenNumbers as you described below. Your mathOperation function will provide differenceBetweenNumbers with these arguments for a and b. Does this make more sense?
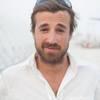
Geert Van Campenhout
7,308 PointsOh I see. Yes it definitely clarifies some things.. Thus is it important that the amount of Int's of both functions are the same (in this case)?
like differenceBetweenNumbers has 2 parameters of type Int "a" and "b" and our mathOperation also has 2 Int parameters (not 3 for instance)
Thank you for your support
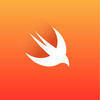
Steven Deutsch
21,046 PointsRight. If you look inside of the mathOperation function, you will see it returns this:
return mathOp(a, b)
What's really happening here is mathOp is replaced with the first argument you pass in, which is differenceBetweenNumbers. Then the values for a and b are passed into differenceBetweenNumbers. So your mathOperation function needs to have two parameters of type Int because that is what will be used inside mathOp.
Looking at it like this might help a bit:
return differenceBetweenNumbers(a, b)
This is what your function is doing under the hood. So you could use ANY other math operation function you create, that uses two parameters a and b. This would look like this:
func sumOfTwoNumbers(a: Int, b: Int) -> Int {
return a + b
}
let sum = mathOperation(sumOfTwoNumbers, 20, 10)
This is why functions are so powerful!
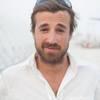
Geert Van Campenhout
7,308 PointsWaw, thanks for your extensive reply! Very helpful!
Geert Van Campenhout
7,308 PointsGeert Van Campenhout
7,308 PointsI see Treehouse didn't attach the instructions for the second part of the task (the part I'm stuck on) so here it goes:
"Using the differenceBetweenNumbers function as an input to mathOperation, compute the difference between any two integers and assign the result to a constant named difference"