Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial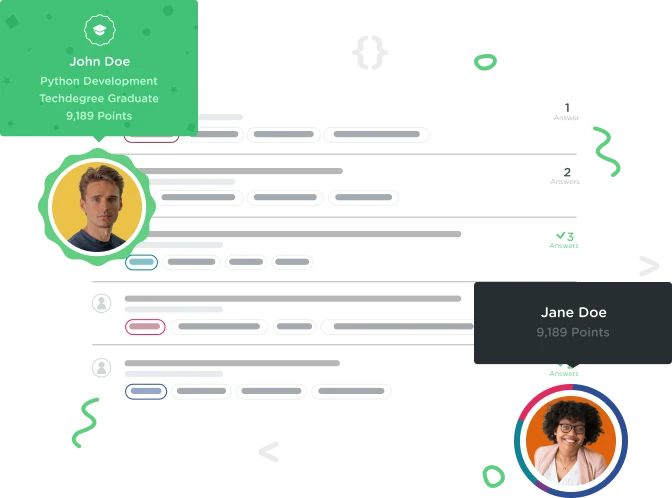

benson sanga
642 PointsStuck on tile game
Second day of coding my whole life stuck here and i would love some help
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
tiles += tile;
}
public boolean hasTile(char tile) {
return tiles.indexOf(tile) != -1;
}
public int getCountOfLetter(char letter){
for (char tile: tiles.toCharArray()){
int number=0;
if (hasTile){
int newNUmber= number++;
}
return newNumber;
}
}
}
// This code is here for example purposes only
public class Example {
public static void main(String[] args) {
ScrabblePlayer player1 = new ScrabblePlayer();
player1.addTile('d');
player1.addTile('d');
player1.addTile('p');
player1.addTile('e');
player1.addTile('l');
player1.addTile('u');
ScrabblePlayer player2 = new ScrabblePlayer();
player2.addTile('z');
player2.addTile('z');
player2.addTile('y');
player2.addTile('f');
player2.addTile('u');
player2.addTile('z');
int count = 0;
// This would set count to 1 because player1 has 1 'p' tile in her collection of tiles
count = player1.getCountOfLetter('p');
// This would set count to 2 because player1 has 2 'd'' tiles in her collection of tiles
count = player1.getCountOfLetter('d');
// This would set 0, because there isn't an 'a' tile in player1's tiles
count = player1.getCountOfLetter('a');
// This will return 3 because player2 has 3 'z' tiles in his collection of tiles
count = player2.getCountOfLetter('z');
// This will return 1 because player2 has 1 'f' tiles in his collection of tiles
count = player2.getCountOfLetter('f');
}
}
1 Answer

Collin Halliday
Full Stack JavaScript Techdegree Student 17,491 PointsHi Benson,
You are on the right track with the use of a for-each loop. The loop will allow your getCountOfLetter method to loop through all of the tile chars in the tile String, but you want to code a way for your method to compare each tile in tiles with the letter passed in as the argument to your method.
It looks like you are attempting to call your hasTile method to accomplish this comparison. In order to call that method, you will need to include parentheses and pass in a tile char as an argument (i.e. if(hasTile(letter))). However, that will not allow you to compare each tile char in the tiles String with the letter parameter. Instead, for each tile in tiles, if the letter parameter of the method is included in the player's tiles, it will increase the tile count, resulting in a count of 6 (the total number of a player's tiles).
To fix this, in the conditional expression of your for-each loop, try setting the letter parameter equal to tile. This will ensure that the counter only increases when a particular tile matches the letter you are testing.
A couple of other issues I noticed. You declare two different variables to keep track of the count--number and newNumber. You only need one variable. You could use two variables if you prefer, but you will want to change the newNumber assignment in the if statement inside your for-each loop. Currently, if the condition in your if statement is true, newNumber is set to number++. Because you have included the ++ on the right side of number, it will not increment until after the variable assignment, so newNumber will be set to 0 the first time the code block in your if statement runs. If nothing else, to keep your code more concise and a little simpler, I would get rid of newNumber and just use number.
You will want to declare and initialize your number variable (i.e. int number = 0;) at the very top of your getCountOfLetter method. Otherwise you will run into scope issues, and your program will not recognize the number variable later in your method. For instance, because you declare your number variable inside the for-each loop, it will not be recognized outside of the for-each loop. Similarly, because you declare your newNumber variable inside the if statement within your for-each loop, it will not be recognized outside of the if statement.
Your method is going to require a return statement, and that statement must be placed outside of the for-each loop. You can then increment your number variable within the for-each loop (i.e. number++), and when it is done looping through all the tiles, your program will exit the for-each loop and return the value of the variable in your return statement (i.e. return number;).
I hope that helps. Best of luck!