Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial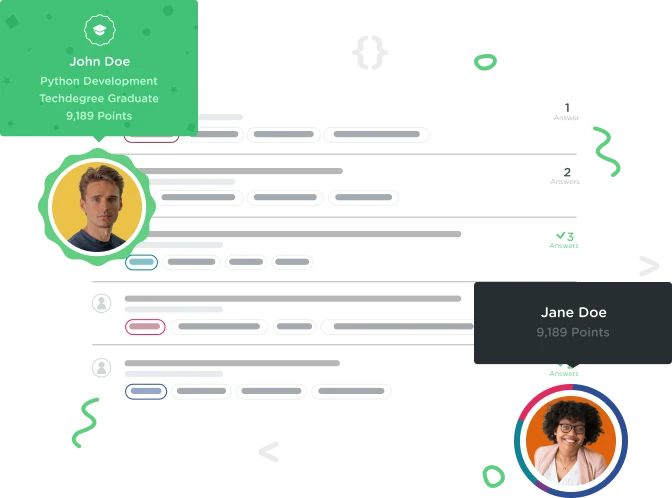
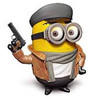
Martin Zarate
10,723 PointsStuck trying to override the Equals(object obj) method. Here is my code:
I'd been trying for a while now and I am just spinning my wheels at this point, any help is greatly appreciated, here is the code:
using System;
namespace Treehouse.CodeChallenges
{
public class VocabularyWord
{
public string Word { get; private set; }
public VocabularyWord(string word)
{
Word = word;
}
public override string ToString()
{
return Word;
}
// this is my code - the one that does not work :(
public override bool Equals(object obj)
{
if ( !(obj is Word) )
{
return false;
}
Word that = obj as Word;
return this == that;
}
}
}
using System;
namespace Treehouse.CodeChallenges
{
public class VocabularyWord
{
public string Word { get; private set; }
public VocabularyWord(string word)
{
Word = word;
}
public override string ToString()
{
return Word;
}
public override bool Equals(object obj)
{
if ( !(obj is Word) )
{
return false;
}
Word that = obj as Word;
return this == that;
}
}
}
1 Answer

andren
28,558 PointsYou seem to have slightly misunderstood the challenge.
The name of the class is VocabularyWord not Word. Word is a property that exists on the VocabularyWord
class.
So the equal method is meant to check if the obj
is a VocabularyWord
instance and then cast it as such if that is the case. Then return true
or false
based on whether the Word
properties of the two objects match up.
Since most of your code is fine besides the naming confusion all you need to do to fix your code is to change all references of Word
to VocabularyWord
, and then to add .Word
to your comparison. Like this:
using System;
namespace Treehouse.CodeChallenges
{
public class VocabularyWord
{
public string Word { get; private set; }
public VocabularyWord(string word)
{
Word = word;
}
public override string ToString()
{
return Word;
}
public override bool Equals(object obj)
{
if ( !(obj is VocabularyWord) )
{
return false;
}
VocabularyWord that = obj as VocabularyWord;
return this.Word == that.Word;
}
}
}
Martin Zarate
10,723 PointsMartin Zarate
10,723 PointsAwesome, thank you. I had it with the VocabularyWord class originally, but I changed it trying to fix the issue. All I was missing was the .Word property call on the return statement. Thank you for the quick response.