Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial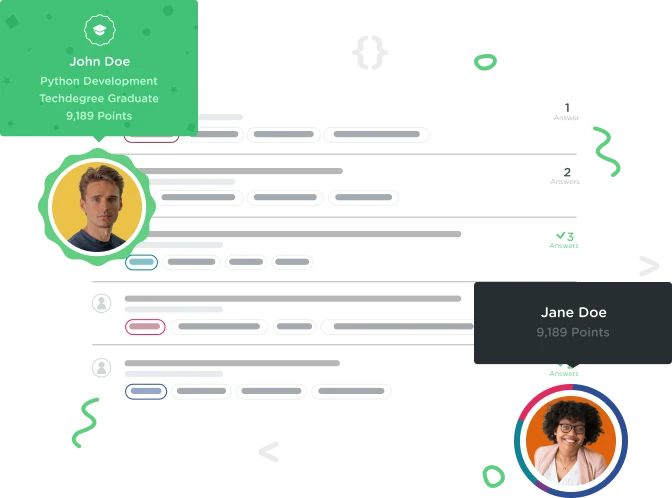

Unsubscribed User
48,988 Pointsstuck with detailsKitchen !?
<?php
class Render {
public static function displayDimensions($size)
{
return $size['length'] . " x " . $size['width'];
}
public function getDimensions()
{
return $this->size;
}
public static function detailsKitchen($room)
{
$room = self::displayDimensions($size->getDimensions());
}
$size->displayDimension('length'=> 12, 'width'=> 14);
echo detailsKitchen($size);
}
?>
3 Answers
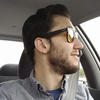
Benjamin Larson
34,055 PointsHere's what I used for my detailsKitchen function:
<?php
public static function detailsKitchen($room) {
return "Kitchen Dimensions: " . self::displayDimensions($room->getDimensions());
}
?>
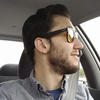
Benjamin Larson
34,055 PointsHi Jens,
Did you get Task 2 to complete with the code you provided? I couldn't get it to pass using associative keys, I have to use numeric indexes:
return $size[0] . " x " . $size[1];
Task 4 makes a big jump in difficulty and number of steps. I'm not sure exactly where you're stuck, but I can provide some hints based on your code. You don't need to define the getDimensions() function at all. As well, you don't need to hard code the 'length'=> 12, 'width'=> 14 values. We're assuming all of that information is being pulled by another file behind the scenes.
This code you have here:
self::displayDimensions($size->getDimensions());
is very close to what you need, but as a return statement. Think through the problem with the above assumptions and let me know if you have any more questions.

Unsubscribed User
48,988 Pointsstill doesn't work, do i have to declare a private variable at the top ?
<?php
class Render {
public static function displayDimensions($size){
return $size[0] . " x " . $size[1];
}
public static function detailsKitchen($room){
$room = self::displayDimensions($size->getDimensions());
return $room;
}
$room->getDimensions(12, 14);
echo Render::displayDimensions($room);
}
?>
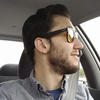
Benjamin Larson
34,055 PointsNope. The code challenge has the dimensions of the room object hard-coded in the background, so you'll need to use:
<?php
$room->getDimensions()
?>
inside your displayDimensions() function. The problem is actually easier than you're probably making it. The entire return statement for detailsKitchen() can be written on one line without assigning anything to the $room object.

Unsubscribed User
48,988 Pointsno idea what i am doing any hint ?
<?php
class Render {
public static function displayDimensions($size){
return $size[0] . " x " . $size[1];
}
public static function detailsKitchen($room){
return self::displayDimensions($size->getDimensions());
}
$room->getDimensions();
echo Render::displayDimensions($room);
}
?>
Unsubscribed User
48,988 PointsUnsubscribed User
48,988 Pointsthanks bro