Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial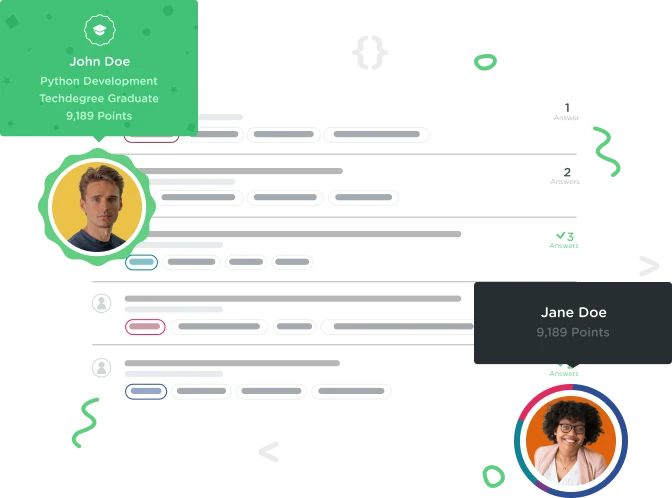

grctniu75rx
6,328 Pointsstuck with returning numbers based on how many times a letter is found in a phrase in Java
"You'll need to use your skills to loop through each of the tiles, use an equality check, and then increment a counter if the tile and letter match. You got this!
Now in your new method, have it return a number representing the count of tiles that match the letter that was passed in to the method. Make sure to check Example.java for some example uses."
To be honest, I have spent way too much time doing this, and after I seem to get far using one possible answer or another, I always end up very close but not complete. At this point I am just wasting time. How would anyone suggest to code this with the shortest possible syntax, as I seem to be over-complicating everything.
See the last method.
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
tiles += tile;
}
public boolean hasTile(char tile) {
return tiles.indexOf(tile) != -1;
}
public int getCountOfLetter(char letter){
}
}
// This code is here for example purposes only
public class Example {
public static void main(String[] args) {
ScrabblePlayer player1 = new ScrabblePlayer();
player1.addTile('d');
player1.addTile('d');
player1.addTile('p');
player1.addTile('e');
player1.addTile('l');
player1.addTile('u');
ScrabblePlayer player2 = new ScrabblePlayer();
player2.addTile('z');
player2.addTile('z');
player2.addTile('y');
player2.addTile('f');
player2.addTile('u');
player2.addTile('z');
int count = 0;
// This would set count to 1 because player1 has 1 'p' tile in her collection of tiles
count = player1.getCountOfLetter('p');
// This would set count to 2 because player1 has 2 'd'' tiles in her collection of tiles
count = player1.getCountOfLetter('d');
// This would set 0, because there isn't an 'a' tile in player1's tiles
count = player1.getCountOfLetter('a');
// This will return 3 because player2 has 3 'z' tiles in his collection of tiles
count = player2.getCountOfLetter('z');
// This will return 1 because player2 has 1 'f' tiles in his collection of tiles
count = player2.getCountOfLetter('f');
}
}
4 Answers
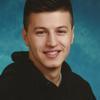
Fahri Can
9,921 PointsIterate with a enhanced for loop through your String tiles and compare it with a IF-Statement, if it is equal with your parameter.
public int getCountOfLetter(char letter){
int count = 0;
for (char temp : tiles.toCharArray()) {
if (temp == letter) {
count++;
}
}
return count;
}
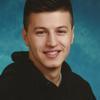
Fahri Can
9,921 PointsI hope this helps

grctniu75rx
6,328 PointsOh! Thank you so much, I kept trying to do it with reusing the char letter in the for loop instead of declaring the new temp, I completely understand it now!

Nirbhay Agarwal
2,852 PointsInstead of adding new temp variable we can use the tile
tile == letter

Mehmet Arabaci
4,432 PointsHi, Okay I struggled with this one a bit too. I understand that the code below is the answer from reading this forum but, I have some questions to understand why
public int getCountOfLetter(char letter) {
int numberCount = 0;
for (char i : tiles.toCharArray()) {
if (letter == i) {
numberCount++;
}
}
return numberCount;
1./ when I first did the code I was testing the char letter which was passed to the method. Why doesn't this work? i.e.
for (letter : tiles.toCharArray()) {
Does this not mean test if the variable letter : [is in] tile.CharArray() ? and that way we could do something like if true numberCount +1?
2./ Secondly obviously seen as the right answer was to use a temp variable, why were we able to define and initialize it within the for loop rather than having to do it the usual way of:
Char i;
and then using it from here?
Thanks

Andrew Winkler
37,739 Pointsi felt like it made more sense using a basic for loop, so here's my code that passed.
public int getCountOfLetter(char letter) {
//set count to zero
int count = 0;
//go through each letter of the tiles with a for loop
for (int i = 0; i < tiles.length(); i++){
//you can only compare one letter at a time so set it to c
char c = tiles.charAt(i);
//compare the singular letter 'c' to the tile submitted as an argument
if ( c == tile){
//if true, add one to count
count++;
}
}
//end for loop and return count
return count;
}

chase singhofen
3,811 Pointsi would have never know to use the temp also, i was putting the loop under and outside of the public int getCountOfLetter(char letter){ method
looking at the example.java didnt help much i was just trying to return a value i.e. return 5, 6, 2 ect. then i tried using count and a value.
i dont understand the syntax errors much but have learned to check my braces {}
Fahri Can
9,921 PointsFahri Can
9,921 PointsYou should use a enhanced for loop