Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial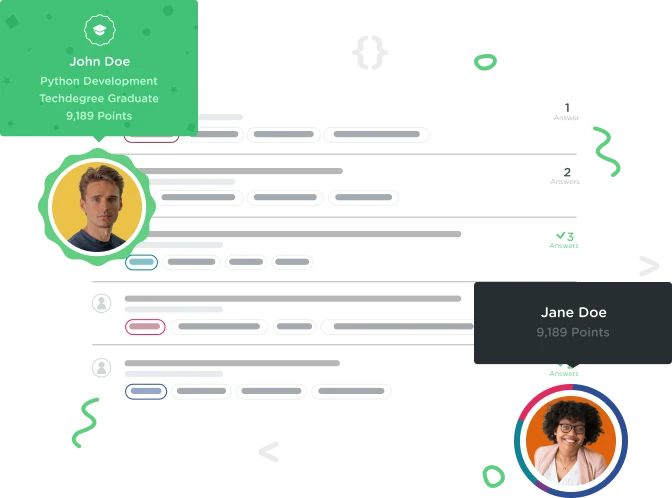
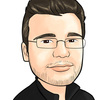
Dani Ivanov
10,732 PointsStudent Record Challenge (my solution)
I just thought to share the code I wrote with the extra challenge of showing results if two or more students match the search query and a message if there is no match. Fun stuff :)
Comments are welcome!
var rawHTML = "";
var arrayNames = [];
var students = [
{name: 'Karolyne Mosby', track: 'Web Design', achievements: 41, points: 6642},
{name: 'Dani Ivanov', track: 'Any', achievements: 999, points: 'Infinity'},
{name: 'John Snow', track: 'Android Development', achievements: 21, points: 1255},
{name: 'Eric Cartman', track: 'Hacking Banks', achievements: 0, points: 0},
{name: 'Kyle Reese', track: 'iOS Development', achievements: 21, points: 7541},
{name: 'Ingrid Homesick', track: 'Front-end Development', achievements: 55, points: 4572},
{name: 'Rowan Akkerman', track: 'Computers for Blonds 101', achievements: 81, points: 7014},
{name: 'John English', track: 'History of Computer Coding', achievements: 11, points: 1012},
{name: 'Jasper Hollande', track: 'Computer Hardware', achievements: 21, points: 1542},
{name: 'Dani Ivanov', track: 'Building web apps', achievements: 11, points: 2563}
];
function print(message) {
var output = document.getElementById('output');
output.innerHTML = message;
}
function searchAndAssign (object) {
var search = prompt('Enter a student name to search or quit to exit');
var student;
var names = [];
if(search.toLowerCase() === 'quit') {
console.log('quit');
return 'quit'
}
for (var i = 0; i < object.length; i++) {
student = object[i];
if(search === student.name) {
console.log('true');
arrayNames.push([student.name, student.track, student.achievements, student.points]);
}
}
if(arrayNames.length <= 0) {
return "empty";
}
return "found";
}
function buildArray () {
for(var i = 0; i < arrayNames.length; i++) {
for(var j = 0; j < arrayNames[i].length; j++) {
if(j === 0) {
rawHTML += '<h2>' + arrayNames[i][j] + '</h2>';
} else if (j >= 1) {
rawHTML += '<p>' + arrayNames[i][j] + '</p>';
}
}
}
}
while(true) {
var answer = searchAndAssign(students)
if (answer === 'found') {
buildArray();
print(rawHTML);
break;
}
if(answer === 'empty') {
print('<h2>Not Found</h2>');
}
if(answer === 'quit') {
print('<h1>Goodbye!</h1>');
break;
}
}