Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial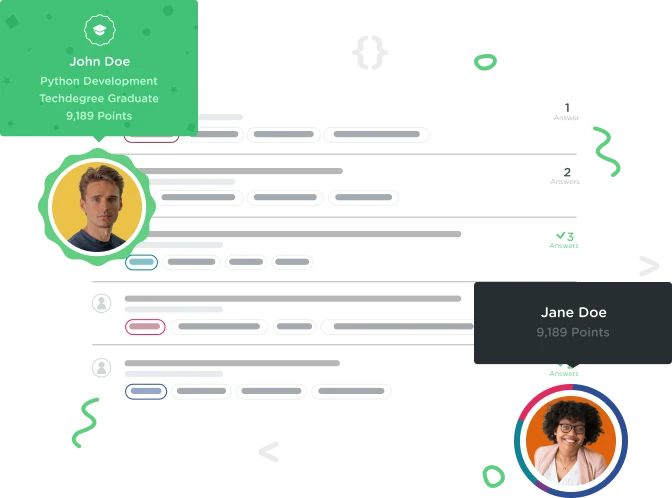
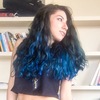
Joslyn Rosbrook
27,259 PointsStudent Record Challenge (Solution)
I finally figured out how to display the extra credit for this challenge (if anyone else was interested in checking their answer)! The user can get information on multiple students with the same name as well as receive a message if their name search doesn't match any names in the array.
var message = '';
var student;
var search;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport(student){
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while(true) {
message = '';
search = prompt("Search student records? Type a student name [John] or type 'quit' to exit.");
if (search === null || search.toLowerCase() === 'quit') {
break;
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (student.name === search) {
message += getStudentReport(student);
}
}
if (message == false) {
message = "<h2>No student named " + search + " was found. Try again.</h2>";
print(message);
} else {
print(message);
}
}
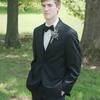
Jamison Imhoff
12,460 PointsI'm probably just blinded by the text, but which section of the code covers the users with the same name issue?

Andrei Fecioru
15,059 PointsThe part where he is able to show students with the same name is in the following section of code:
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (student.name === search) {
message += getStudentReport(student);
}
}
He goes through all the students and, if he finds a match, he appends another HTML-formatted student report to the final output HTML stored in the variable message
. The fact that he appends to the variable message
instead of just setting a new value for it makes this possible (note the use of the +=
operator) .
Andrei Fecioru
15,059 PointsAndrei Fecioru
15,059 PointsIt would be better if you did not rely on the "falsy" boolean value of the empty string. In the last part of the program you do this:
This works because you are not using the "strict equality operator" (i.e. the
===
operator). If you were to use that (which is considered a general good practice from what I understand), the code would no longer work as expected.I would re-write that piece of code in the following manner:
That way you get to be very explicit with the condition you are testing and not rely on the automatic boolean conversion of the
message
string brought in by the simple equality operator (i.e. the==
operator).