Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial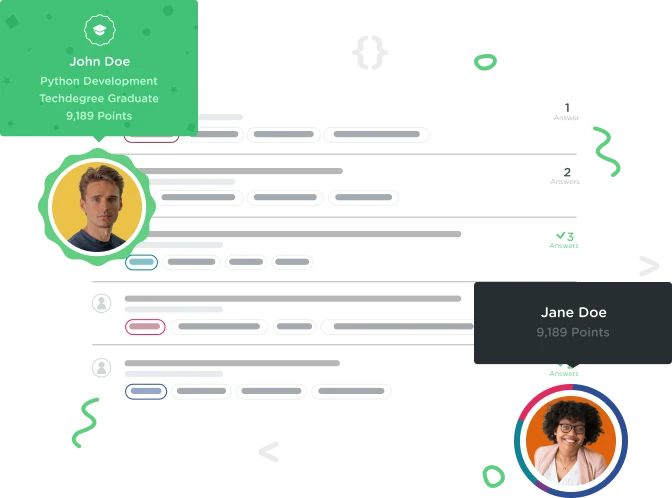

Pete Webb
8,531 PointsStudent Record Search Challenge - My Solution
Any feed back to this solution of the Student Record Search Challenge would be appreciated.
The array here is called students as it is in the introductions to the challenges
const print = message => {
let div = document.getElementById("output");
div.innerHTML = message;
}
let message = "";
let check = true;
do {
let query = prompt(`Search student records: type a name of a student or type "quit" to end`);
for (let i=0; i<students.length; i++) {
if (query.toLowerCase() === students[i].name.toLowerCase()) {
for (let prop in students[i]) {
if (prop === "name") {
message += `<h3>Student: ${students[i].name}</h3>`;
} else {
capitalProp = prop[0].toUpperCase() + prop.slice(1);
message += `<p><strong>${capitalProp}: </strong> ${students[i][prop]}</p>`;
}
}
check = false;
}
}
if (query === "quit") {
alert("You have quit");
break;
} else if (check) {
alert("Please enter a student's name");
}
} while (check)
print(message);
Comments for the code, I thought I'd separate them out.
/* Function: write "message" to HTML element with id "output". */
const print = message => {
let outputDiv = document.getElementById("output");
outputDiv.innerHTML = message;
}
let message = ""; /* initiates message string for print function */
let check = true; /* forces the do-while loop to continuously run unless "check"
variable changes to false */
do {
let query = prompt(`Search student records: type a name of a student or type "quit" to end`); /* continuously prompts user while check = true */
for (let i=0; i<students.length; i++) { /* iterates through the indices of
students array. */
if (query.toLowerCase() === students[i].name.toLowerCase()) { /* returns true
if the user prompt in lower case is strict equal to the
students.name value in lower case for each index of the students array.
We can be flexible with the user input and how the variable is stored in the object.*/
/* so, if true: */
for (let prop in students[i]) {
if (prop === "name") {
message += `<h3>Student: ${students[i].name}</h3>`;
} else {
capitalProp = prop[0].toUpperCase() + prop.slice(1);
message += `<p><strong>${capitalProp}: </strong> ${students[i][prop]}</p>`;
} /* telling it to cycle through the properties in student array index.
If the property/key is strict equals to "name" then we get "Student: (student.name)".
Else, we report eg "Track: Web Design". I decided to change the
property/key name dynamically so it wouldn't appear in all lowercase */
} /* end of for-loop for properties in an object */
check = false; /* since we've found a match of the query in the students
array we can exit the do-while loop by declaring check = false */
} /* end of if-statement */
} /* end of for-loop that iterates through the students array indices */
/* At this point, if the name entered is one of the students.name in the students array then
we know the following will all be false. The value of check = false now too and therefore will
exit the do-while loop. */
/* If the name entered is NOT one of the students.name then: either... */
if (query === "quit") {
alert("You have quit");
break; /*... we break out of the loop if the user types "quit" or... */
} else if (check) {
alert("Please enter a student's name");
} /* ... we haven't found a student's name in the list, check = true, so we tell the user to
enter a student's name. Since check is true we then loop all the way back to the start of the
do-while loop */
} while (check)
print(message); /* updates the HTML to include the elements above if they're there */
Happy to try and answer any questions should anyone be as stuck on this as I was!
2 Answers
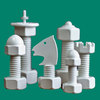
Steven Parker
231,275 PointsGood job.
One suggestion: you might want to check for "quit" before you search the students list for a match with it.

Noah Varner
1,502 Pointsvar message = '';
var student;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
while(true){
let search = prompt('Search for a student\'s name!')
let response = search.toLowerCase()
if(response === 'quit'){
console.log('finished')
break;
}else {
let student = students.find(c => c.name = response)
message += '<h2>Student: ' + student.name + '</h2>';
message += '<p>Track: ' + student.track + '</p>';
message += '<p>Points: ' + student.points + '</p>';
message += '<p>Achievements: ' + student.achievements + '</p>';
}
}
print(message)

Pete Webb
8,531 PointsNice work!
Thank you for showcasing .find()
method, didn't know of that before. Definitely saves looping through and testing each index for the student name. As it returns undefined
if the value is not in the array, you could definitely return something should the name searched not be in the array
I'm wondering why you used var
keyword for some of the variables and let
for the others? For example, you used var students;
at the start only to declare it again with let student = students.find(...);
?
Pete Webb
8,531 PointsPete Webb
8,531 PointsGood idea! I didn't consider the time it would take to search for "quit" before it can break, will amend my code. Thanks!