Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial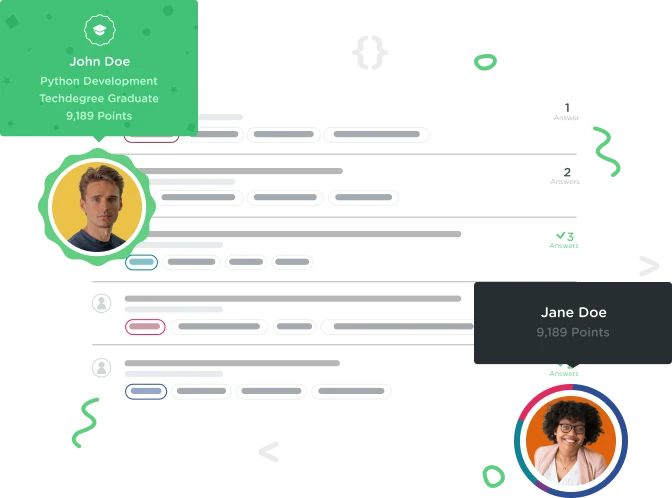
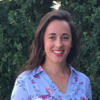
Kortney Field
14,091 PointsStudents are being filtered to top student properly.
When I run the code, I was given davemcfarland as the top student (great). Then I increased the points for kennethlove. I was still getting davemcfarland back. (I saved the code before running). I tried exiting and reopening the workspaces. I then ran it again and was getting craigsdennis back (clearly has the least amount of points). So I closed and opened the workspaces again. I am getting davemcfarland again. (I didn't change anything to the code between tests).
Here is my code. Is there something with my code or something wrong with my workspaces?
from peewee import *
db = SqliteDatabase('students.db')
class Student(Model):
username= CharField(max_length=225, unique=True)
points = IntegerField(default=0)
class Meta:
database = db
students = [
{'username': 'kennethlove',
'points': 14788},
{'username': 'chalkers',
'points': 11912},
{'username': 'joykesten2',
'points': 7363},
{'username': 'craigsdennis',
'points': 4079},
{'username': 'davemcfarland',
'points': 14700},
]
def add_students():
for student in students:
try:
Student.create(username=student['username'],
points=student['points'])
except IntegrityError:
student_record = Student.get(username=student['username'])
student_record.points = student['points']
student_record.save()
def top_student():
student = Student.select().order_by(Student.points.desc()).get()
return student
if __name__ == '__main__':
db.connect()
db.create_tables([Student], safe=True)
add_students()
print("Our top student right now is: {0.username}".format(top_student()))
2 Answers
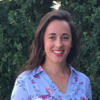
Kortney Field
14,091 PointsThank you. I didn't realize. https://w.trhou.se/evfo3nn1y6
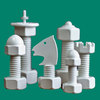
Steven Parker
231,275 PointsI don't understand the part about getting Craig's name, but I think I see the other issue.
The database is loaded from the file "students.db", which already exists in the workspace, and contains names that conflict with those in the "students" list. That causes the "add_students" function to fail, so you just get the results based on what was in the file to start with.
So to see the different results, you just need to delete the old database file before you run the Python program. I gave it a try for confirmation, and it worked.
Steven Parker
231,275 PointsSteven Parker
231,275 PointsYou can share the entire workspace by making a snapshot and posting the link to it.