Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial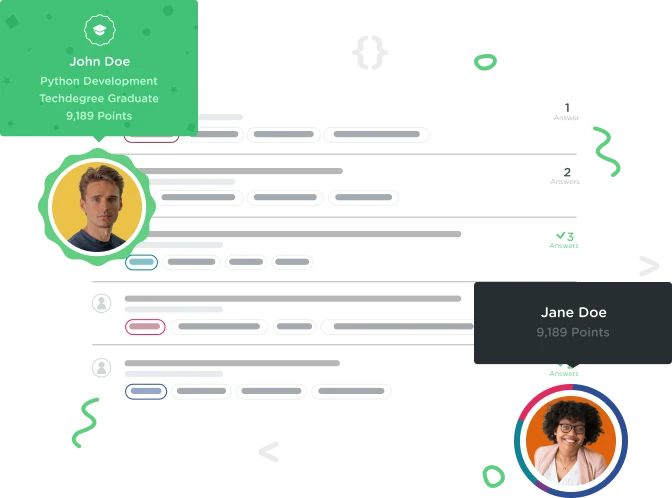
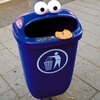
Łukasz Czuliński
8,646 PointsStumped on a JavaScript function
Basically I'm trying to create a list with the ability to add a name on the end. I'm running into two problems.
My first issue is that my function seems to skip over my 'if' statement when I fail to enter a valid string.
My second issue is that push isn't seeming to work as I keep getting this message returned in the console: "*Uncaught TypeError: Cannot read property 'push' of undefined *"
Here's my full code:
var pList = ["Tina V", "Luke B", "Joe T", "Gregor A", "Adria B", "Brad F"];
function addPassenger(pName, pList) {
pName = prompt("Please type in the name of the new passenger.");
if (typeof pName != "string") {
alert("I'm sorry but that is an invalid passenger name.");
return false;
}
else {
pList.push(pName);
alert(pName + " has been added to the passenger list.");
return pList;
}
}
addPassenger();
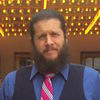
Adam Moore
21,956 PointsThe ' key is the one with the "~" on it, too. The mark is, on my mac, at the bottom of the key. The whole key is located at the top left above my tab key and left of my 1 key.
3 Answers

Riley Hilliard
Courses Plus Student 17,771 PointsSo the issue you had was that you were declaring arguments in your function, however you were not PASSING in the values when you called your function. Also you do not need to pass in any arguments to your function, as everything you appear to be trying to do should be contained in the function itself, or should be effecting the global var 'pList'.
So give this a try and see if this might be what you were trying to figure out? if not let me know and I'll see if I can rework it a bit.
var pList = ["Tina V", "Luke B", "Joe T", "Gregor A", "Adria B", "Brad F"];
function addPassenger() {
var pName = prompt("Please type in the name of the new passenger.");
if (typeof pName != "string") {
alert("I'm sorry but that is an invalid passenger name.");
return false;
} else {
pList.push(pName);
alert(pName + " has been added to the passenger list.");
return pList;
}
}
var logThis = addPassenger();
console.log(logThis);
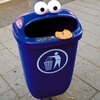
Łukasz Czuliński
8,646 PointsAwesome! Thank you Riley. That seems to do the trick.
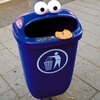
Łukasz Czuliński
8,646 PointsThanks guys. I was pressing the ' by the ", not by the ~.

Riley Hilliard
Courses Plus Student 17,771 PointsGot an answer for you, drafting it up now.
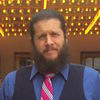
Adam Moore
21,956 PointsThe function addPassenger()
was seeming to take arguments when you declared your function, but you didn't write any of them in the call of the function. However, that doesn't really matter because all of the variables inside the function are either defined inside the function or they reference their global declaration that was made outside of the function. The arguments for the function are only necessary if, when the function is called, arguments are passed in with the function call, which isn't necessary because you have prompts within your code that serve to set the variables inside your function. Or at least I'm pretty sure that's how it works.
Hope this helps!
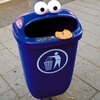
Łukasz Czuliński
8,646 PointsThanks Adam! I see my errors in the declare/call.
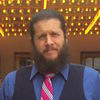
Adam Moore
21,956 PointsAlso, since prompt()
only returns a string, anything you put in the field will be converted into a string. The only way the error message is shown is if the Cancel button is pushed. Otherwise, you can type in anything and the prompt function will turn it into a string and add it to your array. I'm not exactly sure what function you could use to make sure that that isn't the case (like if a number was entered), but I'm sure there is one; I just haven't looked it up yet.
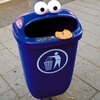
Łukasz Czuliński
8,646 PointsAh yes. Of course the prompt return will most always be a string.
I'm just reading now about the function that checks if a string contains specific characters; that should work better for what I'm trying to do.
Riley Hilliard
Courses Plus Student 17,771 PointsRiley Hilliard
Courses Plus Student 17,771 PointsI can help you format your code, so I'll go ahead and edit your post real quick.