Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial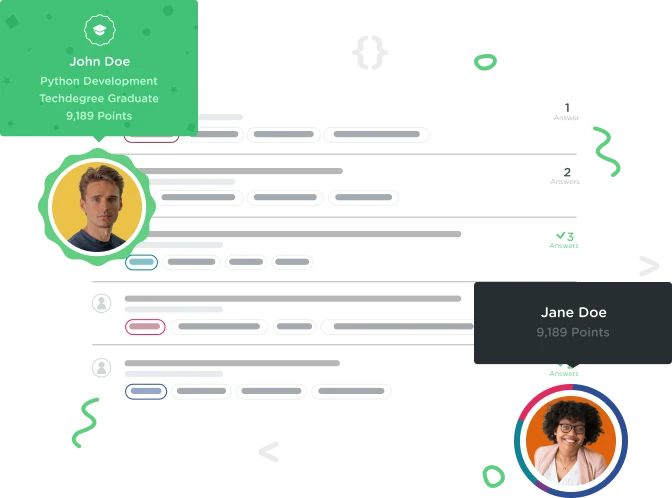

Ra Bha
2,201 PointsStumped on how to write the code to determine average length of the frog tongue after the for loop
Is it possible to declare a variable averageLength and define it as sum of the lengths of the tongues of the frogs in the frogs array then divide this by the index of the frogs array? I read somewhere that you cannot perform math on arrays or their indexes.
var averageLength = sum TongueLengths /frogs[i].TongueLength
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double GetAverageTongueLength(Frog[] frogs)
{
for(int i = 0; i < frogs.Length; i++)
{
Frog frog = frogs[i];
}
var sum = ______;
int averageTongueLength = sum / frogs[i];
return averageTongueLength
}
}
}
namespace Treehouse.CodeChallenges
{
public class Frog
{
public int TongueLength { get; }
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
}
}
2 Answers
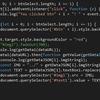
Damien Bactawar
8,549 PointsHere is my solution (I've tested it and it works).
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double GetAverageTongueLength(Frog[] frogs)
{
int Total = 0;
for(int i = 0; i < frogs.Length; i++)
{
Total += frogs[i].TongueLength;
}
return (Total / frogs.Length);
}
}
}
// frogs[i].TongueLength;
// TongueLength is an integer and is an attribute of the Frog object.
// In this case it is the only attribute of the Frog object.

Ra Bha
2,201 PointsHi Damien, thank you! Your explanation helps a lot. I am going to mark your answer best answer here.
I also came up with code that I think solves the problem (not quite yours though) and I would appreciate if you take a look at it as well: link
Thanks again
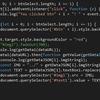
Damien Bactawar
8,549 PointsI took a look and left a message.
Ra Bha
2,201 PointsRa Bha
2,201 PointsThanks, Damien. I see how it would work.
Aside from the solution, your comments below your solution code indicate that Frog is an object. How did you determine that - isn't Frog a class?
Damien Bactawar
8,549 PointsDamien Bactawar
8,549 Points"Aside from the solution, your comments below your solution code indicate that Frog is an object. How did you determine that - isn't Frog a class?"
As I understand it, a class is like a blueprint for making an object. When you instantiate a class you are creating an instance of the object (I used the cake recipe to make a single cake). Inside the for-loop you tried to do this (Frog frog = frogs[i];). However in this example there is no need for this. The method GetAverageTongueLength accepts a array parameter named frogs which is of type Frog[]. "frogs" is an array of Frog objects but it is also an object which has already been created and is passed to the method. The for-loop iterates through the array of Frog objects and.... well you know the rest. You were thinking along the right track.
Anyway I hope this helps and please remember to mark/accept my answer "up" if it did!