Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial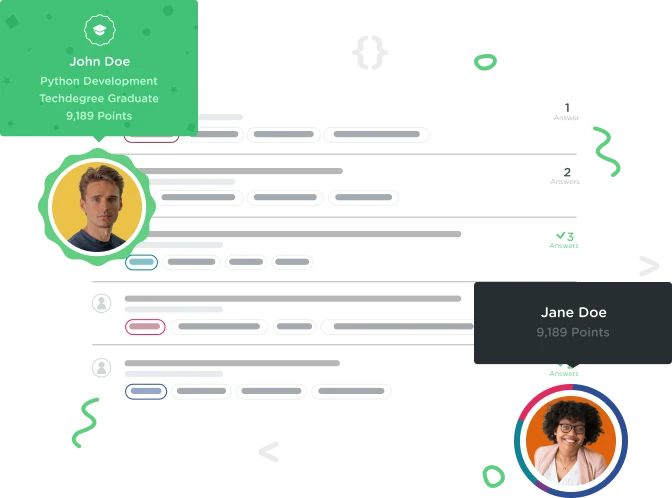
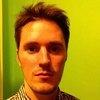
Brendan Whiting
Front End Web Development Techdegree Graduate 84,736 PointsStumped on loopy
Code:
def loopy(items):
for item in items:
if item[0] == "a":
continue
else:
for member in item:
print(member)
Error: "Bummer! Didn't find the right items being printed."
5 Answers
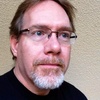
Chris Freeman
Treehouse Moderator 68,423 PointsSince the first code block of the if
ends in a continue, there is no need for the else
:
# Task 1 of 1
# Same idea as the last one. My 'loopy' function needs to skip an
# item this time, though.
# Loop through every item in 'items'. If the current item's index 0
# is the letter "a", 'continue' to the next one. Otherwise, print
# out every member of items.
def loopy(items):
for item in items:
if item[0] == 'a':
continue
print(item)
Since loops have an implied continue
at the end, this can be rewritten as:
def loopy(items):
for item in items:
if item[0] != 'a': # <-- reversed test to not 'a'
print(item)
continue # <--can be omitted as last statement in loop

Michael Norman
Courses Plus Student 9,399 PointsThe issue is with the loop in your else block. It is stating that for each letter in item, print that letter. For the challenge you just want to print the the entire word if it does not start with 'a'
def loopy(items):
for item in items:
if item[0] == "a":
continue
else:
print(item)

Gary Gibson
5,011 PointsThis is the one that worked for me.

Jonathon Mohon
3,165 PointsWhen I did this I wrote it exactly like your first example Chris and it wouldn't work. When I switched to using the != it worked. I have no idea why one would work and not both.
Also I typed this in a script and called loopy using the word "assassin" thinking it would omit the first 'a' but print the second one but it omitted both a's. I thought this since the second 'a' was in a different index. Can someone clarify what this is supposed to do please?

Marvin Calderon
710 PointsI also had this same issue.
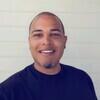
Eric Fernandez
Python Development Techdegree Student 3,008 PointsAfter reading the question, I understood as coding the following:
def loopy(items):
# Code goes here
for things in items:
if "a" == 0:
continue
else:
print(things)
break
could some explain to my if my logic, syntax, or both are wrong if so why and what can i do to better my understanding.thanks you
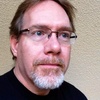
Chris Freeman
Treehouse Moderator 68,423 PointsWhat does the 0 in condition "a" == 0
represent?
As written the 0 is simply the integer value zero. To look at the first element or item of things
use index notation: "a" == things[0]

timoleodilo
1,438 Pointsdef loopy(items): for things in items: if (things[0] == "a"): continue else: print(things)
this is what I did and it worked. becarefull for syntax error. it took me 30 min to realize it. good luck
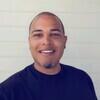
Eric Fernandez
Python Development Techdegree Student 3,008 PointsChris Freeman I did not know how to reference the index. i thought that was the correct way to say " a is positions 0"