Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial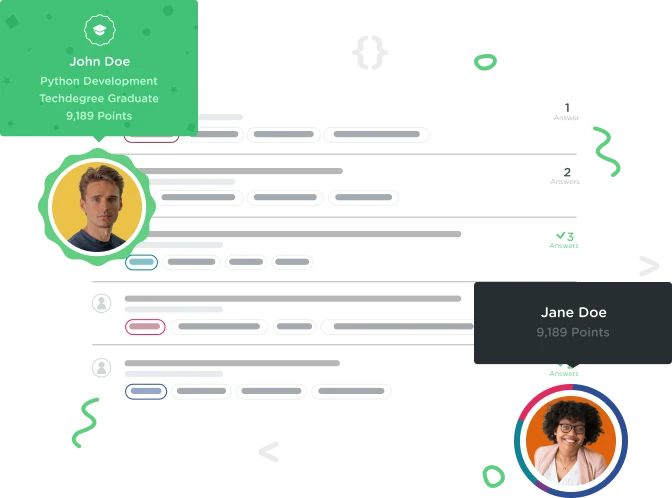

Shane Meikle
13,188 PointsStyle the label of a select box when select box is hovered?
<label for="blah">Size</label>
<select id="blah">
<options all up in here>
</select>
What I want to do is have the label text "SIZE" to change color when a user opens up the dropdown menu on the select box. It should then change back when the user moves on to something else.
Any help or a point in the right direction would be greatly appreciated, thanks!
5 Answers
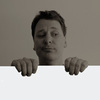
Sean T. Unwin
28,690 Points/* You can use both or either. Depends on what you want or need */
#sizeme:focus + label,
#sizeme:hover + label {
color: red;
}
/* to be explicit and to let readers know what to expect */
#sizeme:blur + label {
color: black;
}
As David Omar pointed out, you will need to refactor your HTML so that the label is after the <select>
. See below.
<div>
<select id="sizeme">
<option>Woah</option>
</select>
<label id="sizeme-label" for="sizeme">Size:</label>
</div>
I have updated the example to include what you require. Scroll towards the bottom of the CSS to see (it's the same as I pasted at the top of this post).
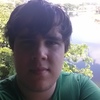
David Omar
5,676 PointsYou will need to use the :focus pseudo class to achieve your affect. Also you coded your html wrong, it should be:
<select id="blah">
<option>all up in here</option>
</select>
<label for="blah">Size</label>
I placed the label after the select element because I don't know how to select an element before another one.
select:focus + label{
color: red;
}
The :focus pseudo class targets an element that is activated by your mouse or keyboard. The + signifies an immediate sibling of the select element which is why I dropped the label element below the select element. If you want it the other way around I'm sure you can make it so by using the float css property.

Shane Meikle
13,188 PointsThank you for responding. I am not sure if I understand what you mean by the HTML is coded wrong, though.
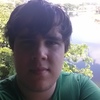
David Omar
5,676 Points<label for="blah">Size</label>
<select id="blah">
<options all up in here>
</select>
you messed up the option element
<option>all up in here</option>

Shane Meikle
13,188 PointsOh, I see what you meant. The:
<options all up in here>
was meant as a placeholder for all the <option></option> elements and not as actual code.
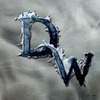
Hugo Paz
15,622 PointsTry something like this:
<select id="blah">
<option>1</option>
<option>2</option>
<option>3</option>
<option>4</option>
</select>
<label id="label" for="blah">Size</label>
both elements need to be inside the same parent element. At this stage the label needs to come after the select element due to the + selector we are going to use.
Your css should be something like this
#label{
float:left;
}
#blah:hover + #label{
color:red;
}
Now depending on your remaining code, you might need to adjust the css. The float:left will put your label on the left of the select element. If this solution does not do it for you, you can try using jquery.

Shane Meikle
13,188 PointsThanks for the response, I will test it out and give it a shot.
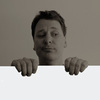
Sean T. Unwin
28,690 PointsEdit: I misread the question. I have updated the pen and posted another answer elsewhere in this thread.
It's as simple as increasing the font-size
on the select, but there will be aesthetic issues and possible breakage of your layout.
I have created a working example on Codepen.io for you to see some options to use to minimize layout issues and some aesthetics.
The code is below as well for the lazy ;-p
<div>
<label id="sizeme-label" for="sizeme">Size:</label>
<select id="sizeme">
<option>Woah</option>
</select>
</div>
/* container */
div {
width: 8em;
height: 2em;
margin: 1em;
}
#sizeme-label {
margin-right: 0.15em;
margin-top: 0.1em;
/* the following is a hack so the label does not move */
float: left;
}
#sizeme {
width: 5em;
height: 1.3em;
}
/* including :active here so snap back
* won't happen until mouse is moved
*/
#sizeme:hover,
#sizeme:active {
font-size: 1.25em;
}

Shane Meikle
13,188 PointsThank you for the response, but it is the label I was looking to have styled while having the selectbox hovered. (ie. change the color of size from black to red while hovered over the selectbox.
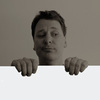
Sean T. Unwin
28,690 PointsYeah sorry about that I misread your post. I'll post another answer.

Shane Meikle
13,188 PointsAfter playing around with the advice given above and other random attempts, I went ahead and used a cheap 'fix' of sorts. I surrounded the label/select pair in their own divs and then using a child selector to target the label within the div when it is being hovered.