Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial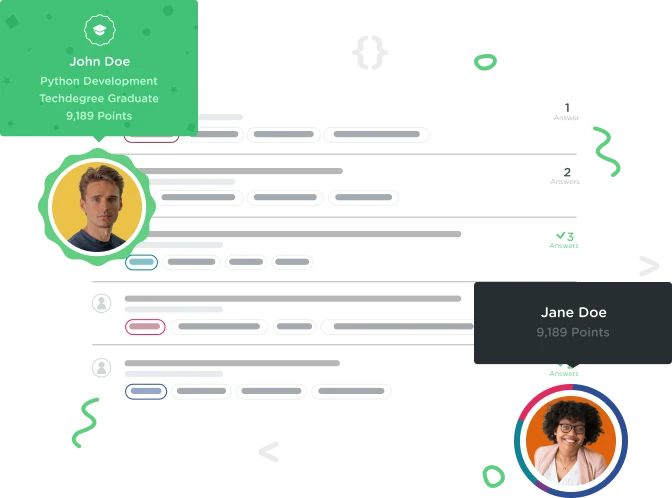
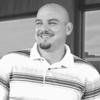
Ryan Breece
4,160 PointsSubclass Question. The code runs. What am I missing?
I added what they are asking for. The code seems to be running as I can see the new value change when I create an Instance of move"up". Am I missing something?
In the editor you've been provided with two classes - Point to represent a coordinate point and Machine. The machine has a move method that doesn't do anything because most machines are motionless. Your task is to subclass Machine and create a new class named Robot. In the Robot class, override the move method and provide the following implementation. If you enter the string "Up" the y coordinate of the Robot's location increases by 1. "Down" decreases it by 1. If you enter "Left", the x coordinate of the location property decreases by 1 while "Right" increases it by 1. Note: If you use a switch statement you can use the break statement in the default clause to exit the current iteration.
class Point {
var x: Int
var y: Int
init(x: Int, y: Int){
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: String) {
print("Do nothing! I'm a machine!")
}
}
// Enter your code below
class Robot: Machine {
override init() {
super.init()
}
override func move(direction: String) {
switch direction{
case "Up" : location.y + 1
case "Down" : location.y - 1
case "Left" : location.x - 1
case "Right" : location.x + 1
default : break
}
}
}
let player = Robot()
player.location = Point(x: 2, y: 2)
player.move("Up")
print(player.location.y)
3 Answers
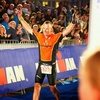
Steve Hunter
57,712 PointsHi Ryan,
You've done the hard bit here! But, your increment and decrement execution isn't quite right. You're not assigning the values back into the x or y components. You can do that long-hand, or just use the ++ or -- operators. And you don't need to mess with the init
method. It should look something like:
class Robot: Machine {
override func move(direction: String){
switch direction{
case "Up" : location.y++
case "Down" : location.y--
case "Left" : location.x--
case "Right" : location.x++
default : break
}
}
}
I hope that helps.
Steve.

Bronson Barrett
4,624 Points//There seems to be an error in the initial template code already added by Team Treehouse.
class Point {
var x: Int
var y: Int
init(x: Int, y: Int){
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: String) {
print("Do nothing! Im a machine!") /*I deleted the apostrophe in Im (I'm), and my code compiled... Don't know if anyone had this issue, but it wouldn't let me complete the challenge until I made this change. */
}
}
// Enter your code below
class Robot: Machine {
override init() {
super.init()
}
//an alternative way to increase and decrease the x,y values is with the += and -=
override func move(direction: String) {
switch direction{
case "Up" : location.y += 1
case "Down" : location.y -= 1
case "Left" : location.x -= 1
case "Right" : location.x += 1
default : break
}
}
}
[MOD: edited code blocks. SH]
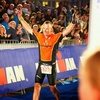
Steve Hunter
57,712 PointsHi Bronson,
Deleting the apostrophe will make the code format in colours. Alternatively, you can escape the apostrophe character with a backslash - your code will pass the challenge either way. Deleting the apostrophe did not make your code pass; it just made the colour format appear.
Steve.
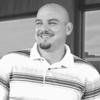
Ryan Breece
4,160 PointsYes, makes sense. I was thinking to myself, "How is this returning?" But I because I am new to this still, I started trying to find solutions in the init or the method return. Thank you alot.
Ryan Breece
4,160 PointsRyan Breece
4,160 PointsThank you for the quick response. I have been reading and watching youtube videos for a while on this.
Ryan Breece
4,160 PointsRyan Breece
4,160 PointsFor Learning, would you mind showing me the long hand version of making this happen.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsNo problem!
Saying
location.y + 1
doesn't affectlocation.y
. You need to assign something back into it. Going long would look likelocation.y = location.y + 1
. Using the increment operator works just the same.Steve.
Steve Hunter
57,712 PointsSteve Hunter
57,712 Pointsi6ze5srftzgi8o5iu46z5etr
6,931 Pointsi6ze5srftzgi8o5iu46z5etr
6,931 Pointsclass Robot: Machine { override func move(_ direction: String) { switch direction { case "Up": location.y += 1 case "Down": location.y -= 1 case "Left": location.x -= 1 case "Right": location.x += 1 default: break } } }