Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial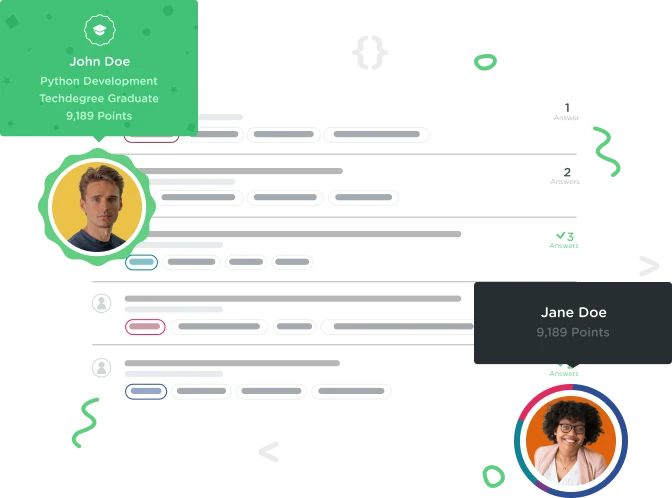

shane reichefeld
Courses Plus Student 2,232 PointsSubClass Whats wrong? and why
explanation
class Person {
let firstName: String
let lastName: String
init(firstName: String, lastName: String) {
self.firstName = firstName
self.lastName = lastName
}
func getFullName() -> String {
return "\(firstName) \(lastName)"
}
}
// Enter your code below
class Doctor: Person {
override init(firstName: String, lastName: String) {
self.firstName = "Dr."
self.lastName = lastName
}
}
let someDoctor: String = Doctor(Sam , lastName: Smith)
1 Answer
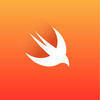
Steven Deutsch
21,046 PointsHey shane reichefeld,
You're close, but the challenge is asking you to override the getFullName() method and not the initializer of the Person class. This overriden version of the method will return "Dr. (lastName)" instead of its original "(firstName) (lastName)" implementation.
Let's take a look at this below:
class Person {
let firstName: String
let lastName: String
init(firstName: String, lastName: String) {
self.firstName = firstName
self.lastName = lastName
}
func getFullName() -> String {
return "\(firstName) \(lastName)"
}
}
// Enter your code below
class Doctor: Person {
override func getFullName() -> String {
return "Dr. \(lastName)"
}
}
// When calling the initializer you need to provide the argument names
// Also make sure what you are passing in is what is required
// You need to be passing in two Strings here
let someDoctor = Doctor(firstName: "Sam", lastName: "Smith")
someDoctor.getFullName()
Good Luck