Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial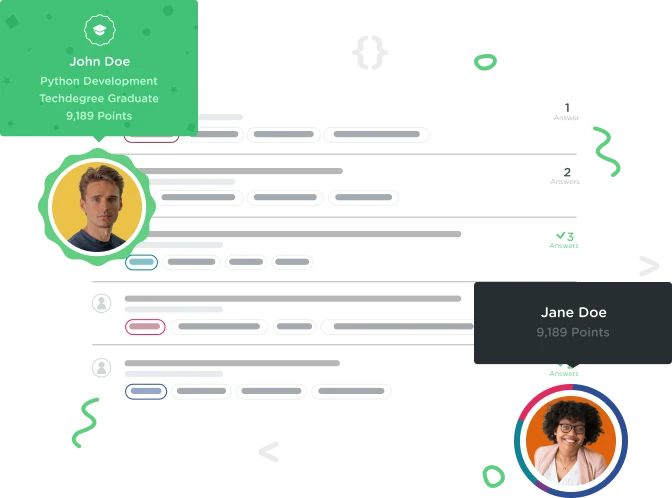
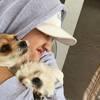
Carlos Marin
8,009 PointsSubclassing a list
"Now I want you to make a subclass of list. Name it Liar.
Override the len method so that it always returns the wrong number of items in the list. For example, if a list has 5 members, the Liar class might say it has 8 or 2.
You'll probably need super() for this.:"
I am getting a "Maximum recursion depth exceeded" when I try to get the length. What does this mean????
class Liar(list):
def __init__(self, *args, **kwargs):
self = super().__init__(*args, **kwargs)
return self
def __len__(self):
return len(self)+1
3 Answers
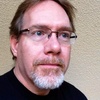
Chris Freeman
Treehouse Moderator 68,423 PointsWhen the code len(some_obj)
is encountered, it calls some_obj.__len__()
if this method uses len(some_obj)
the method will continuously call itself (recursion) until the maximum level (or depth) is reached.
By using super().__len__()
you can get a true length then alter it in the Liar class.
Post back if you need more help. Good luck!!!
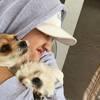
Carlos Marin
8,009 PointsHey Chris! I was confused, it took me a while but It's starting to add up now. I just solved the code challenge named "frustration".
I am a bit puzzled with the current class object I have now. why is my list being cleared?
:: below is my code ::
class Liar(list):
def __init__(self, *args, **kwargs):
self = list.__init__(*args, **kwargs)
return self
def __len__(self):
return super().__len__()+1
input: books = ['cooking', 'fishing']
input: len(Liar(books))
output: 1
input: Liar(books)
output: []
input: len(books)
output: 0
Thanks a ton! You're the best!
- Carlos A. Marin
[MOD: added ```python formatting -cf]
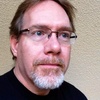
Chris Freeman
Treehouse Moderator 68,423 PointsInteresting question. I didn’t notice it before. The answer may surprise you: the list created by Liar
will always be empty
- the method
__init__
is not expected to return anything. It only modifies Theself
that points to the instance - the statement
list.__init__(
anything)
will always returnNone
- Assignment to variable that has the same name as a parameter creates new variable local to that method instead of changing the original object referenced by the parameter. By assigning
self
, the valueself
becomes local to the method.
Since nothing special is being performed by Liar.__init__
it could be removed:
class Liar(list):
def __len__(self):
return super().__len__()+1
books = ['cooking', 'fishing']
print(books)
print(len(Liar(books)))
print(Liar(books))
print(books)
print(len(books))
print(books)
#output
[‘cooking’, ‘fishing’]
3
[‘cooking’, ‘fishing’]
[‘cooking’, ‘fishing’]
2
[‘cooking’, ‘fishing’]
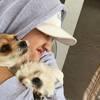
Carlos Marin
8,009 PointsThanks for coming thru Chris! I feel loads better now! :D