Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial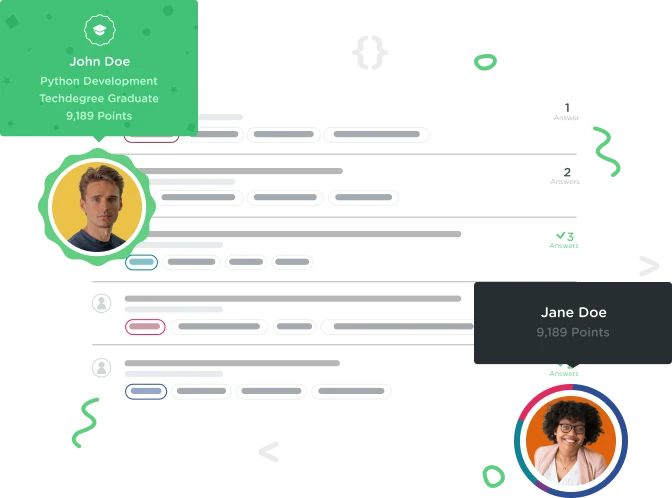

Craig Watson
27,930 PointsSubmit button not disabling with jQuery?
Hi everyone,
I'm on with the jQuery Basics and one of the sections requires some interactivity adding to a form. The part I am stuck on is the very last stage where my code should now disable the submit button when the given criteria of 8 characters and matching confirm passwords are not met.
Here's my code
//Problem: Hints are shown even when form is valid!
//Solution: Hide and show them at appropriate times!
var $password = $("#password");
var $confirmPassword = $("#confirm_password");
//Hide Hints
$("form span").hide();
//Functions
function $isPasswordValid(){
return $password.val().length > 8;
}
function $arePasswordsMatching(){
return $password.val() === $confirmPassword.val();
}
function $canSubmit() {
return $isPasswordValid() && $arePasswordsMatching();
}
function $passwordEvent(){
//Find out if password is valid
if($isPasswordValid()) {
//Hinde hint if valid
$password.next().hide();
} else {
//Else show hint
$password.next().show();
}
}
function $confirmPasswordEvent() {
//Find out if password and confirmation match
if($arePasswordsMatching) {
//Hide hint if matched
$confirmPassword.next().hide();
} else {
//Else show hint
$confirmPassword.next().show();
}
}
function $enableSubmitEvent(){
$("#submit").prop("disabled", !$canSubmit());
}
//When event happens on password input
$password.focus($passwordEvent).keyup(passwordEvent).keyup($confirmPasswordEvent).keyup($enableSubmitEvent);
//When event happend on confirmation input
$confirmPassword.focus($confirmPasswordEvent).keyup($confirmPasswordEvent);
$enableSubmitEvent();
All help will be much appreciated as this is bugging me a lot!
Thanks
1 Answer
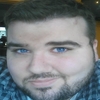
Marcus Parsons
15,719 PointsHey Craig Watson,
Good to see ya again! haha :) I took a look at your code, and it looks like you're just missing a couple more attached events to your $password and $confirmPassword fields. Here are the ones you should have attached:
//When event happens on password input
$password.focus($passwordEvent).keyup($passwordEvent).focus($confirmPasswordEvent).keyup($confirmPasswordEvent).keyup($enableSubmitEvent);
//When event happens on confirmation input
$confirmPassword.focus($confirmPasswordEvent).keyup($confirmPasswordEvent).keyup($enableSubmitEvent);
If you have any questions about those extra events, I'm here to help!
Craig Watson
27,930 PointsCraig Watson
27,930 PointsHey Marcus!
The code I missed on is clearly one of the most important parts of the video .... Who knows where the mind goes in these situations .. haha.
Thanks for the help Marcus, I am now debugging another part of the code as for some unreasonable reason the confimPasswordEvent does not seem to be executing.....
Thanks Again Craig
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsTry putting a () after the function call in the if-statement of your $confirmPasswordEvent function. It's not calling the function as it should, and I do believe that should fix your error.
Anytime, Craig! I know how it is when you get tired or what not, and your mind goes other places haha