Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial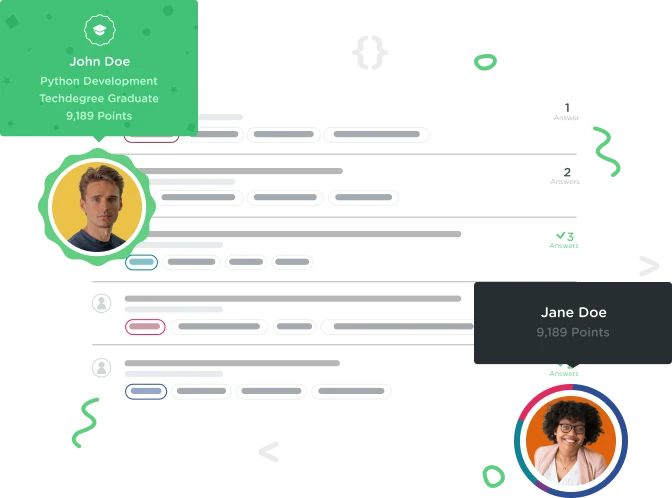
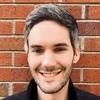
Jesse Vorvick
6,047 PointsSuggestions for my code?
Here is my code. It works, but it turns out it is a bit complex compared to Dave's. Perhaps I should have kept it more simple? Tell me what I can do differently!
/*
Quiz asks 5 questions.
After the player inputs the answers,
The player is shown how many he got right
and ranked by receiving a gold, silver, or
bronze crown, if any.
*/
// Questions
var Answer1 = prompt('What is the word for a group of owls?');
var Answer2 = prompt('What color is my cat litter tub?');
var Answer3 = prompt('How many buttons are on the side of my mouse?');
var Answer4 = prompt('How many cups in a gallon?');
var Answer5 = prompt('How many pounds in a ton?');
if (Answer5 === '2,000') {
Answer5 = '2000'
}
var score = 0
// Check for correct answers, add to score
if ( Answer1.toUpperCase() === 'PARLAMENT' ){
score += 1
}
if ( Answer2.toUpperCase() === 'BLUE' ) {
score += 1
}
if ( Answer3 === '12' || Answer3.toUpperCase() === 'TWELVE' ) {
score += 1
}
if ( Answer4 === '8' || Answer4.toUpperCase() === 'EIGHT' ) {
score += 1
}
if ( Answer5 === '2000' || Answer5.toUpperCase() === 'TWO THOUSAND') {
score += 1
}
alert('Your final score is ' + score);
/*
Display:
1. 5 correct = Gold crown
2. 3-4 correct = Silver crown
3. 1-2 = Bronze crown
4. 0 = Nothing
*/
if ( score === 5 ){
document.write ('<p> You get a gold crown! </p>' );
} else if ( score < 5 && score > 2 ) {
document.write ('<p> You get a silver crown! </p>' );
} else if ( score < 3 && score > 0 ) {
document.write ('<p> You get a bronze crown! </p>' );
} else {
document.write ('<p> Refresh the page to try again! </p>' );
}
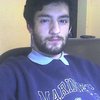
Dario Bahena
10,697 PointsHere is a different take on this code. The key here DRY (Do not Repeat Yourself) All of the questions are stored in an array the answers as well. You do not need to change the type from string to number with parseInt or anything like that because it is unnecessary.
let score = 0;
const questions = [
'What is the word for a group of owls?',
'What color is my cat litter tub?',
'How many buttons are on the side of my mouse?',
'How many cups in a gallon?',
'How many pounds in a ton?'
];
const correctAnswers = [
'PARLAMENT', 'BLUE',
['TWELVE', '12'], ['EIGHT', '8'],
['2000', 'TWO THOUSAND']
];
// loop through the questions array and prompt that question.
// the prompt method ALWAYS returns a string
let currentAnswer;
for (let i = 0; i < questions.length; i++) {
currentAnswer = prompt(questions[i]) // store the user response in a variable
.toUpperCase() // turn the string to uppercase
.replace(',', '') // remove commas
.trim(); // remove left and right white space
if (currentAnswer === correctAnswers[i]) { // check if the answer is the same as the one in your answer list with the same index
score++;
} else if (currentAnswer && correctAnswers[i].includes(currentAnswer)) {
// checks if the answer is not a blank string then checks if the answer is in the list of proper answers like ['TWELVE', '12']
// to avoid manual logical checks
score++;
}
}
alert(`Your final score is ${score}`);
// function expression returning a string
const message = medal => `<p> You get a ${medal} crown! </p>`;
// store score logic in array. this returns a list with booleans you can
//scores indexes correspond to [gold, silver, bronze]
const scores = [score === 5, score < 5 && score > 2, score < 3 && score > 0];
// array of medals available
const medals = ['gold', 'silver', 'bronze'];
// indexOf method finds the first occurrence in an array. So here we look for the first true
const medal = scores.indexOf(true);
// a simple ternary expression (if and else in one line) to present the data
// indexOf method returns -1 if something is not found
medal > -1 ? document.write(message(medals[medal])) : document.write('<p> Refresh the page to try again! </p>')

Sarah Yung
1,070 PointsI am keen to know if Dario's method is the best practice in coding although we haven't learned using for loops and arrays.
anthony amaro
8,686 Pointsanthony amaro
8,686 Pointsremember when using numbers you have to use 'parseInt' keyword
you can change this lines as well, the first line is only going to run if the player got 5 answer correct, if not, then is going to check the second line if the player got less than or equal to 4. if not. then is going to run the next line, if the player got less then or equal to 2.
i hope this helps