Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial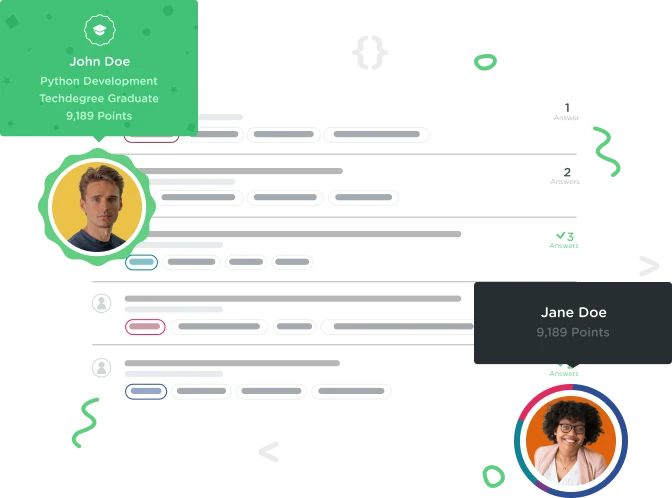

Gary Butler
1,588 Pointssum += many[i]; What is the purpose of the "i" at the end of many in the statement?
I think I understand the loop and how it works mostly but wondering why we need to add the [i] at the end of many?Appreciate it if someone can further explain the purpose of this.
4 Answers

Joseph Taralson
1,737 PointsHi Gary,
I don't blame you for being confused. I understand For Loops quite well, and Doug's explanation is extremely confusing, even for me. Here's the best way to think about it:
The variable "i" is going to change 3 times, once each time it goes through the For Loop. The first time through, i = 0. When the loop starts, it adds sum (which equals 0, as initialized in the beginning) plus many[i] (in this run through the loop, many[1] = many[0], or the first integer initialized up top, 2). Therefore, sum += many[i] is 2.
NOW, the loop has ran 1 time, and sum now equals 2. As we state in our for loop, we auto-increment "i", making "i" now equal 1. Since this is still less than 3, the loop runs again. Sum +=many[i] now translates to: 2 += many[1]. Many[1] is the 2nd integer initialized above, or 4. 2 + 4 equals 6, so the second line prints 6.
NOW, the loop has ran twice, and sum now equals 6. We auto-increment "i", making "i" now equal 2. Since this is still less than 3, the loop runs again. Sum +=many[i] now translates to: 6 += many[2]. Many[2] is the 3rd integer initialized above, or 8. 6 + 8 equals 14, so it prints 14.
Once this run through the loop is done, it auto-increments "i" to 3. Since "i" is no longer < 3, the loop quits and ends the program. Hope this helps clear it up for you.

Kevin Marshall
3,561 PointsThe i is the value that changes when you set up a loop so that i there is selecting the ith index of many. When you create a loop you may still want to refer to the number of times you are going through the loop so you use the variable you set the loop up with to refer to some item within it.

Gary Butler
1,588 Points(have amended my comment to have the all the code below.) Right ok so does the many need to reference to the i value in order to make the loop work or just there incase you want to output the value of i in the printf?
include <stdio.h>
int main() {
// insert code here...
int many[] = {2,4,8};
int sum = 0;
for (int i=0; i < 3; i++) {
sum += many[i];
printf("sum %d\n", sum);
}
return 0;
}

Kevin Marshall
3,561 PointsI don't know for sure without looking at the whole piece of code. It likely does if it is adding to the list many a running sum which it looks like given what you have posted.

Humza Choudry
237 Pointsyou broke it down perfectly. Thank you Joseph Taralson.

toanh tran
9,083 PointsThe explaination was great and I now understand. I was scratching my head at first.
Gary Butler
1,588 PointsGary Butler
1,588 PointsHi Joseph
Thank you for this breakdown. Its greatly helped me get my head around it. I see now i is just for a reference for the code for how many loops are happening and not adding to the equation of the sum.
Much appreciated