Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial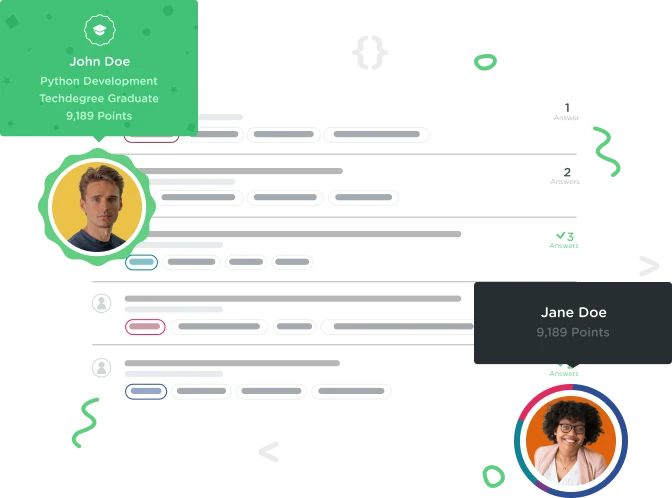

sandeep Singh
381 PointsSuper()
when we are already in subclass which means its going to inherit all the characteristics or the attributes then why Super function Please explain with real life example !!!
2 Answers
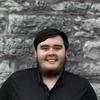
Michael Hulet
47,912 Pointssuper()
is really useful to call a method on a superclass of an object. This is particularly useful when you want to override a method of a superclass of your object, but add onto the superclass's implementation instead of replace it entirely.
This is a somewhat contrived example, but let's say you have a Person
object that holds a first_name
and last_name
and has a method called full_name()
that gives back the full name of the person.
class Person():
def __init__(self, first_name, last_name):
self.last_name = last_name
self.first_name = first_name
def full_name(self):
return self.first_name + " " + self.last_name
It's customary as a sign of respect to refer to a doctor as "Dr. <# full_name #>", but as the Person
object stands now, it treats everybody the same. We can make a subclass called Doctor
to fix that.
class Doctor(Person):
def full_name(self):
return "Dr. " + super().full_name()
Now if we make a Person
object for me, calling full_name()
will just give you my name, but if you make a Doctor
object for my doctor, it'll refer to my doctor properly.
>>> me = Person("Michael", "Hulet")
>>> me.full_name()
'Michael Hulet'
>>> my_doctor = Doctor("Kristen", "Christensen")
>>> my_doctor.full_name()
'Dr. Kristen Christensen'

Andrew McLane
3,385 PointsGreat example, thanks!
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsNice example!