Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial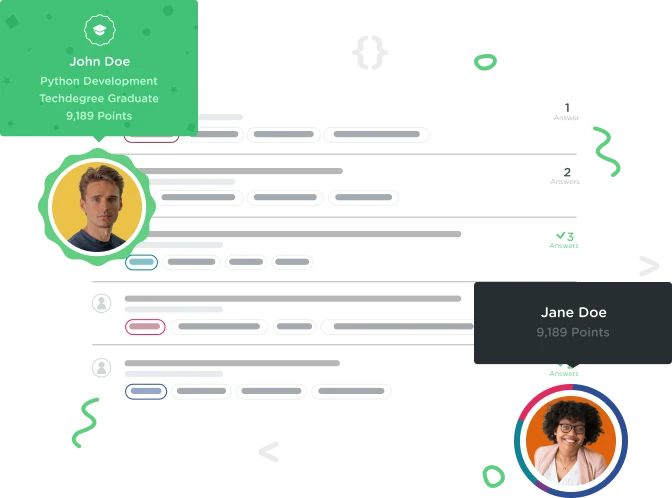

anthony pizarro
1,983 Pointssuper()
i don't understand super(), what is it doing exactly, and it seems like im repeating myself when writing def add_item inside class sortedinventory why are we not using item in this challenge and when should i use it ?
class Inventory:
def __init__(self):
self.slots = []
def add_item(self, item):
self.slots.append(item)
class SortedInventory(Inventory):
def add_item(self, item):
super().add_item(item)
1 Answer
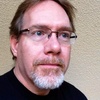
Chris Freeman
Treehouse Moderator 68,423 PointsGood question! The process for calling a class instance method goes as follows:
Say you are looking for the add_item
method in SortedInventory
:
- look in the method
add_item
in the name space of the localSortedInventory
instance - If not found, look in the each parent class listed in the inheritance list. In this case,
Inventory
But what if you want the behavior of Inventory.add_item
but want a bit more in the local version. You could do one of two things:
- copy
add_item
completely intoSortedInventory
then make the changes you need. This isn't Pythonic because if the original method inInventory
was changed then the changes wouldn't be reflected in the copied method. - some how still be able to call both the local and the inherited methods. This is where
super()
helps.
Since SortedInventory.add_item
exists, the method Inventory.add_item
cannot be directly accessed from the local instance. The super()
function provides the solution. Its basic function is to shift execution to the parent's version of the method, then return to execution in the local version. Without the super()
only the local method would execute.
Looking at the point you are at in your code through Task 2, there is really no difference to having the add_item
in SortedInventory
or not at all since the local method only calls super()
then "returns". [side note: no return
is the same as return None
.] Without the SortedInventory.add_item
method, the parent's method would have been called as a matter of course.
It's Task 3 where the advantage of using super()
becomes evident. Additional code can be executed after the parent method returns back to local method execution. In fact, sometime you may want to run code before the super() [before the parent's method], or both before and after the super() [if you need some pre- and post-processing]
The parameter item
is needed so it can be passed along to the parent's method since the parameter list for both the methods (a.k.a., their signatures) need to match.
Post back if you need more help. Good luck!
Ravi Kiran
2,196 PointsRavi Kiran
2,196 PointsThe reason you find this hard to understand is beacuse super() is confusing . Don't fret , everyone stumbles on this one.
Let me try with an example. You have a parent class : Inventory You have a child which is inherited off the parent class : SortedInventory
Imagine your friend gave you a better parent class called SuperInventory and you want to use that like this :
class SortedInventory(SuperInventory):
Imagine all the changes you would have to make in the child class to do that , With super() you don't have to make any changes as long as the caller/callee classes have the same argument pattern. There are other uses for super() too. You will understand as you move along.
Hope this helps !