Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial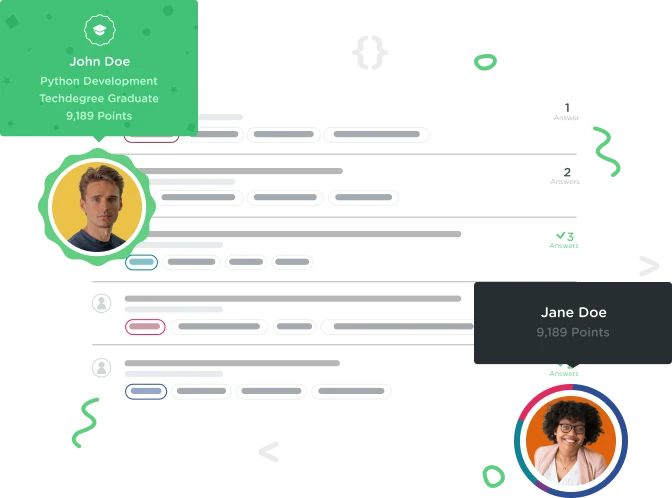

Benjamin McCarthy
2,002 PointsSuper Basic AI program (responds to user entering "hi")
Just getting to grips with concepts so attempted to build a low level scripted chat AI, basically to respond to inputs with if else commands and remember some what a user has said through Let values previously to adapt responses - however struggling to get the basics of using entered text to change the Let values so when the function is called to write to the page it writes the robotResponseVar based on "textbox" value (and how many times a user has said hi)
any help massively appreciated
<head>
<script>
let userInput = document.getElementById("textbox").value;
let userInputLower = userInput.toLowerCase();
let saidHi = 0;
let robotResponseVar = "what?";
if (userInputLower == "hi" && saidHi < 1) {
robotResponseVar = "Oh Hello There";
saidHi += 1;
}else if (userInputLower == "hi" && saidHi > 0) {
robotResponseVar = "Hi again";
saidHi += 1;
}else if (userInputLower == "hi" && saidHi > 3) {
robotResponse2Var = "haha you keep saying that";
saidHi += 1;
}
function writeToPage(){
let robotResponse = robotResponseVar;
let txt = document.createTextNode(robotResponse);
let p = document.createElement("p");
let div = document.getElementById("div");
p.appendChild(txt);
div.appendChild(p);
}
</script>
</head>
<body>
<div id="div">
<input type="text" id="textbox" value="" />
<input type="submit" id="btnClick" value="Click" onclick="return writeToPage();"/>
</div>
</body>
1 Answer
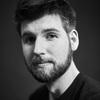
Pavlo Morgun
18,688 PointsI changed the code a little bit to avoid console errors.
<head>
<script>
let userInput;
let saidHi = 0;
let robotResponseVar = "what?";
function checkInput(string) {
if (string == "hi" && saidHi < 1) {
robotResponseVar = "Oh Hello There";
saidHi += 1;
}else if (string == "hi" && saidHi > 0) {
robotResponseVar = "Hi again";
saidHi += 1;
}else if (string == "hi" && saidHi > 3) {
robotResponse2Var = "haha you keep saying that";
saidHi += 1;
}
}
let robotResponse;
function writeToPage(){
userInput = document.getElementById("textbox").value.toLowerCase();
checkInput(userInput);
robotResponse = robotResponseVar;
let txt = document.createTextNode(robotResponse);
let p = document.createElement("p");
let div = document.getElementById("div");
p.appendChild(txt);
div.appendChild(p);
}
</script>
</head>
<body>
<div id="div">
<input type="text" id="textbox" value="" />
<input type="submit" id="btnClick" value="Click" onclick="return writeToPage();"/>
</div>
</body>
Benjamin McCarthy
2,002 PointsBenjamin McCarthy
2,002 PointsHero thanks for your help!