Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial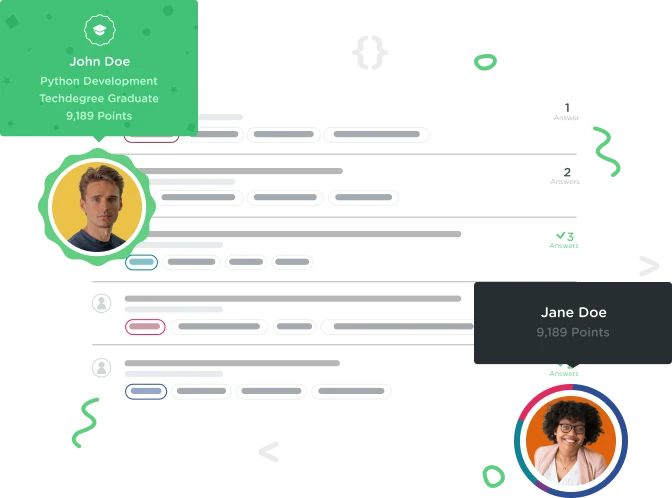

mike sanford
2,389 PointsSuper conditional challenge problem
I keep getting this
Bummer! Hmm. Looks like there is at least one logical OR operator -- that's the || symbols. Those test if just one of the conditions are true.
Here is the code. I thought if I made money ===9 && today === 'friday' that would make the condition true and run that operation.
var money = 9;
var today = 'Friday'
if ( money >= 100 || today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 || today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 || today === 'Friday' ) {
alert("Time for a movie");
} else if (money === 9 && today ==='Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
var money = 9;
var today = 'Friday'
if ( money >= 100 || today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 || today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 || today === 'Friday' ) {
alert("Time for a movie");
} else if (money === 9 && today ==='Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers
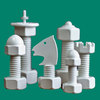
Steven Parker
230,274 PointsYou don't need to change any of the money tests. But you do need to change the way the tests are being combined.
The or operator ( || ) is true if either test is true. You want to change the operator so the expressions are true only if both tests are true. You want each if statement to test the money and the day. Fix that on each line with two tests.
The final test for just the day only needs to change from inequality to equality, you don't need to add another money test to it.
I'm guessing with these hints you can get it without an explicit spoiler.
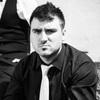
Billy Bellchambers
21,689 PointsYou were close with that you were trying here.
But all you needed to do was change the if statements from || (or) to && (and) so that the passing conditions needed both statments to be true rather than just one.
and lastly with the "It's Friday, but I don't have enough money to go out" change the if statement to give that statement when only the Day var is met.
You can find the correct code below.
var money = 9;
var today = 'Friday'
if ( money >= 100 && today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 && today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 && today === 'Friday' ) {
alert("Time for a movie");
} else if ( today === 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
Hope that helps.
Happy coding.

mike sanford
2,389 PointsThank you Billy. What seemed so difficult now makes total sense.
mike sanford
2,389 Pointsmike sanford
2,389 PointsThank you for your quick reply. After reading your response I can see that I was not understanding the principal of the test. Once you broke it down it was easy enough. It is takeing me more time then I thought to wrap my head around the J.S. but once I see the process it makes it clear. Again thank you. The community with Treehouse is truly superb.