Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial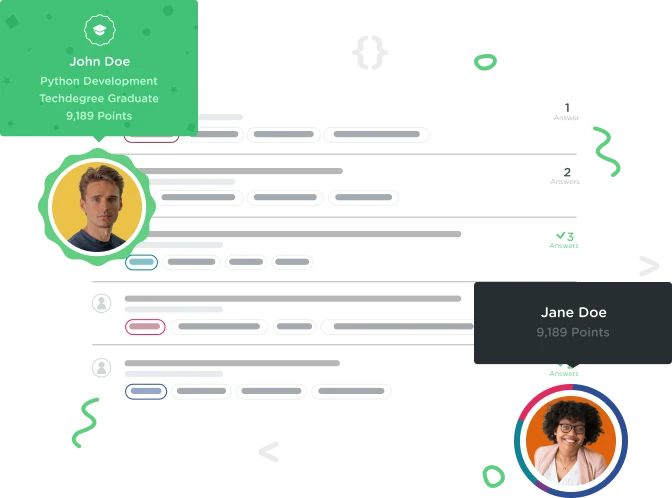

ryan smith
687 PointsSwallowing errors? I don't get what he means.
I don't quite understand @ 2:30 what he means by
except:
It will swallow all errors? I remember him saying that if you wrote
except ValueError:
then if someone got a
TypeError
it wouldn't be caught. So wouldn't the
except:
be useful since any random instance of some error would be caught instead of the term he used "swallowing" whatever that is?
3 Answers
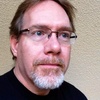
Chris Freeman
Treehouse Moderator 68,454 PointsGreat question! The except block is used to handle errors from the try block. When an exception class is provided, the except block will handle only that exception type. You can have multiple except blocks for a try statement.
If an exception is not handled, the error is automatically reraised up the call chain. If the calling function doesn't handle it, it gets reraised again, and so on until the top level where the stacktrace of errors is displayed from each reraising function and the program stops.
If no exception class is listed on the except
line, then all errors will be handled by this except block. This effectively "swallows"/consumes/hides all errors preventing any error from being reraised and handled or seen outside of the current local block.
Post back if you need more help!

katinkahesselink
2,392 PointsYou are right. If you use *except: *, all errors get caught.
This seems great. However, it lacks precision. It does not tell you (the developer) what went wrong. This is why it is generally advised to go for specific except blocks. That way you know what went wrong, and can target your response accordingly.

Mykiel Horn
4,014 PointsWhen he says swallows, the code will take in any input(numbers, words, ect) that the user inputs. So if there is no except block the user can input whatever they want and the code will take it(swallow) and the developer(aka you) will have to deal with a bunch of inputs that you didn't want in the first place. I hope I explained that correctly! Good luck!!
ryan smith
687 Pointsryan smith
687 PointsI don't understand the whole line "If an exception is not handled, the error is automatically reraised up the call chain. If the calling function doesn't handle it, it gets reraised again, and so on until the top level where the stacktrace of errors is displayed from each reraising function and the program stops." Reraising, Stacktrace, handling... could you possibly simplify the terms for me? Also wouldn't it be good if all errors are handled... then you can find the error your code is having in the try block? Isn't this limited only to the code within the try block?
Chris Freeman
Treehouse Moderator 68,454 PointsChris Freeman
Treehouse Moderator 68,454 PointsThere is a good overview in the docs on errors
It is good that all errors be handled because any unhandled error crashes the program.
I misused the term reraise slightly. Reraising is catching an error in an
except
block, but then explicitly raising it again with araise
command.Handling an error is simply resolving the error in a controlled manner. In Python this is done using try/except statements.
When an unhandled exception occurs the current execution stops and the error is passed to the code calling this function. The error passing upward continues until the error is properly handled or the top level is reached. At the top level, the Python interpreter dumps a stacktrace which listed the error, where it occurred, and all the layers passed through when the error was raised. Scanning through the stacktrace helps debug the issue.
The main purpose of using a try block is to catch the anticipated errors usually surrounding events external to your code (bad user input, filehandle input/output issues) and occasionally inside the code for missing dict keys or type conversion errors.
An unexpected error should not be handled by default so it can be raised all the way to the top where a stacktrace is produced and the code can be debugged. If the unexpected error was "swallowed" with a catch-all
except:
then no debugging occurs because the error gets hidden.An example of reraising an error: