Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial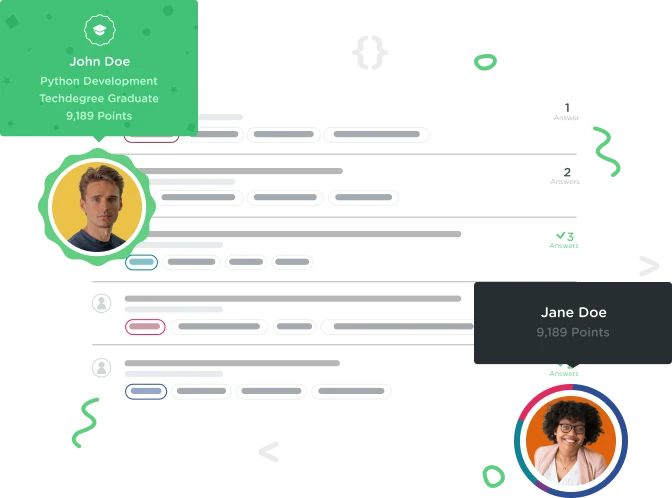
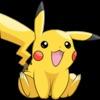
Sean Loke
14,659 PointsSwapHead by Chris - do not understand it
class PezDispenser { private String characterName;
public PezDispenser(String characterName) { this.characterName = characterName; }
public String getCharacterName() { return characterName; }
public String swapHead(String characterName) { String originalCharacterName = this.characterName; this.characterName = characterName; return originalCharacterName; } }
public class Example {
public static void main(String[] args) {
System.out.println("We are making a new PEZ Dispenser"); PezDispenser dispenser = new PezDispenser("Donatello"); System.out.printf("The dispenser is %s %n", dispenser.getCharacterName() ); String before = dispenser.swapHead("Darth Vader"); System.out.printf("It was %s but Chris switched it to %s %n", before, dispenser.getCharacterName()); }
}
Anyone can help me break down step-by-step the swapHead part by chris? Thanks!
2 Answers

andren
28,558 PointsSure
String originalCharacterName = this.characterName;
This creates a new variable called originalCharacterName
that stores what is currently stored in the characterName
field/member variable ("Donatello" in the example above) that is defined at the top of the class. The this
keyword has to be used to help Java distinguish the field/member variable characterName
from the characterName
parameter that is passed in to the function.
this.characterName = characterName;
This sets the field/member variable characterName
to the value of the characterName
parameter, the characterName
parameter will contain whatever string you passed in as the first argument to the method. Which is "Darth Vader" in the example above.
return originalCharacterName;
This simply returns the value stored in the originalCharacterName
variable that was created earlier.
So to summarize, the method creates a variable to hold the current character name, then it changes the character name to one you supplied to the method, then it returns the old name.
If you have any further questions or feel confused by a part of my explanation then feel free to reply to this post with your questions, I'll answer anything I can.

Cassi Emerson
1,910 PointsHi Andren - is it because String before = dispenser.swapHead("Darth Vader"); is creating a new instance of the variable dispenser?

andren
28,558 PointsNo, that line does not create a new instance. All it does is run the swapHead
method within the dispenser
object and then assign the result to the before
variable. It does not do anything beyond that.
The swapHead
method changes the characterName
field on the dispenser to whatever name you pass into it and then returns the old name. That means that dispenser.characterName is now equal to whatever you passed in to the swapHead
method, and before
is equal to whatever the characterName
was before the name change.
Sean Loke
14,659 PointsSean Loke
14,659 PointsThank you! Could you explain this bit as well in the example.java file?
String before = dispenser.swapHead("Darth Vader"); System.out.printf("It was %s but Chris switched it to %s %n", before, dispenser.getCharacterName()); }
andren
28,558 Pointsandren
28,558 PointsString before = dispenser.swapHead("Darth Vader");
This line calls the
dispenser.swapHead
method and then stores the value it returns into thebefore
variable. Since thedispenser.swapHead
returns the name the dispenser had before it was swapped you end up with thebefore
variable containing the old name of the dispenser.So the line both runs
dispenser.swapHead
(which means that thecharacterName
within the dispenser changes) and assigns the value it returns to thebefore
variable.System.out.printf("It was %s but Chris switched it to %s %n", before, dispenser.getCharacterName()); }
This prints out a formatted string. The
%s
symbols are replaced by the string values you provide to theprintf
method, in this case the first%s
is replaced by the contents of thebefore
variable and the second%s
is replaced by the value thatdispenser.getCharacterName()
returns.So you end up with the first
%s
being replaced by the old dispenser name since that was stored in thebefore
variable on the line above, and the second%s
ends up containing the current dispenser name since that is what thedispenser.getCharacterName()
returns.