Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial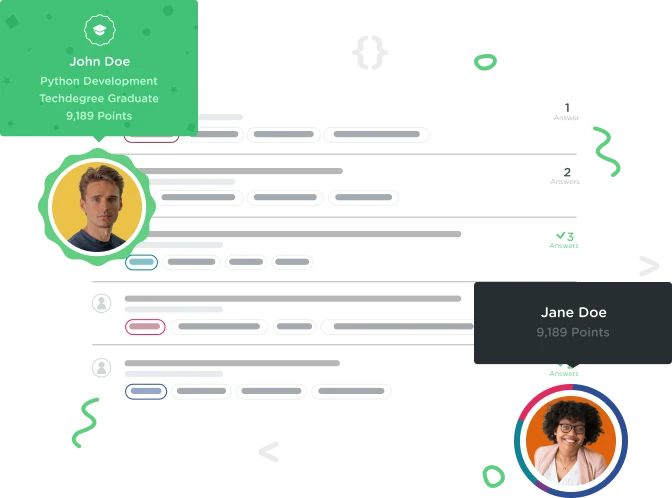
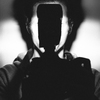
julienclemencon
1,559 PointsSwift 2: replacing ?.toInt() with ?.int()
In Swift 2 toInt() got replaced by int() and this works:
var testString1 = "1" // -> "1"
Int(testString1) // -> 1
var testStringA = "A" // -> "A"
Int(testStringA) // -> nil
However if I replace toInt() in the code with int() I get this error:
if let culprit = findApt("101")?.toInt() { // 'toInt()' is unavailable: Use Int() initializer
sendNoticeTo(aptNumber: culprit)
}
if let culprit = findApt("101")?.int() { // Value of type 'String' has no member 'int'
sendNoticeTo(aptNumber: culprit)
}
What am i doing wrong, or why isn't this working?
Thank you!
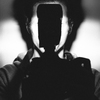
julienclemencon
1,559 PointsHere is all my code, if I change it to if let culprit = findApt("101") as Int I get the error 'String?' is not convertible to 'Int'
Thank you James Lambert!
//: Swift Functions and Optionals - Optionals - Optional Chaining
import UIKit
func sendNoticeTo (aptNumber aptNumber: Int) {
print("Message send")
}
func findApt (aptNumber : String ) -> String? {
let aptNumbers = ["101", "202", "303", "404"]
for tempAptNumber in aptNumbers {
if ( tempAptNumber == aptNumber) {
return aptNumber
}
}
return nil
}
/*
if let culprit = findApt("505") {
if let aptNumber = Int(culprit) {
sendNoticeTo(aptNumber: aptNumber)
}
}
*/
if let culprit = findApt("101") as Int { // Error: 'String?' is not convertible to 'Int'
sendNoticeTo(aptNumber: culprit)
}
let testString1 = "1" // -> "1"
Int(testString1) // -> 1
let testStringA = "A" // -> "A"
Int(testStringA) // -> nil
3 Answers
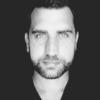
Jhoan Arango
14,575 PointsHello:
Because of the changes to Swift, we want to do this code a bit different.
if let culprit = findApt("101") { // We give a string value to culprit
sendNoticeTo(aptNumber: Int(culprit)!) // Then we can change it to Int.
}
This will be the easiest walk around solution.
Good luck ! :)
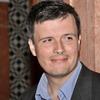
Brian Patterson
19,588 PointsCouldn't the Swift 2.0 videos have been released sooner. As I feel that we as customers are in no mans land. I understand they are being released mid October. Isn't this leaving it a bit late.

Christian Dangaard
3,378 PointsThank you, this also worked for me.

Onur Odabasi
2,151 PointsHi,
With swift 2.0 it became: if let culprit = Int(findApt("101")!){
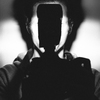
julienclemencon
1,559 PointsThis works only if the aptNumber is in your array, otherwise you wil get an error code.

Mahdi Pedramrazi
2,505 Pointsthis will fail when you add invalid apt number
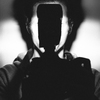
julienclemencon
1,559 PointsOk, Thank you Onur and Jhoan!
It worked :)
James Lambert
Courses Plus Student 14,477 PointsJames Lambert
Courses Plus Student 14,477 PointsWith out seeing the rest of what your doing, it should be: let culprit = findApt("101") as Int
Still, you souldn't declare a variable inside an "if" conditional statement. That's going to through an error.