Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial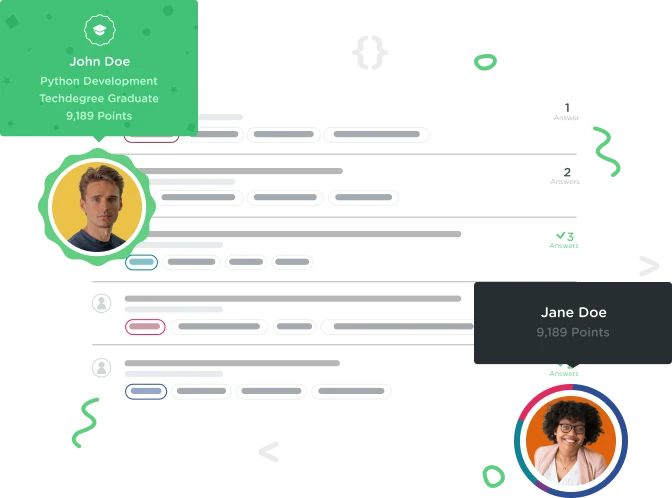

Ary de Oliveira
28,298 PointsSwift 2.0
In the editor, I have provided a class named Vehicle. β¨Your task is to create a subclass of Vehicle, named Car, that adds an additional stored property numberOfSeats of type Int with a default value of 4. β¨Once you've implemented the Car class, create an instance and assign it to a constant named someCar.
I tRY hARD BUT NO...
class Vehicle {
var numberOfDoors: Int
var numberOfWheels: Int
init(withDoors doors: Int, andWheels wheels: Int) {
self.numberOfDoors = doors
self.numberOfWheels = wheels
}
}
class VehicleCar {
let numberOfSeats: Int
let: someCar
}
// Enter your code below
3 Answers
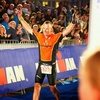
Steve Hunter
57,712 PointsHi Ary,
Here's how that would look:
class Car: Vehicle {
var numberOfSeats: Int
override init(withDoors doors: Int, andWheels wheels: Int){
self.numberOfSeats = 4
super.init(withDoors: doors, andWheels: wheels)
}
}
let someCar = Car(withDoors: 4, andWheels: 4)
We start by declaring that Car
is a sub-class of Vehicle
. We add its unique property, the seats integer, then create the init
method. This sets the unique property first (default value of 4 required), then calls the init
method of the super class, Vehicle
.
Make sense?
Steve.
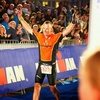
Steve Hunter
57,712 PointsDominic,
Yep, this works; my presumed errors were "correct", so here's my attempt at solving your requirement:
class Car: Vehicle {
var numberOfSeats: Int
init(withDoors doors: Int, andWheels wheels: Int, andSeats seats: Int){
self.numberOfSeats = seats
super.init(withDoors: doors, andWheels: wheels)
}
}
let someCar = Car(withDoors: 4, andWheels: 4, andSeats: 4)
Steve.
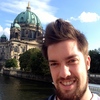
Dominic Bloor
7,857 PointsThanks so much for your help Steve!!!!!! :-)
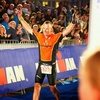
Steve Hunter
57,712 PointsHey, no problem!
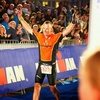
Steve Hunter
57,712 PointsHi Gregory,
I think the call to super
needs to be last, either explicitly or otherwise, as on completing that chain (there could be numerous super class chains) the instance must be complete. Execution shouldn't continue so you do your 'local' setup work, then pass the construction up the chain of super
.
That's my understanding of it anyway!
Steve.
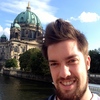
Dominic Bloor
7,857 PointsHi Steve
If we wanted the NumberOfSeats to come up as a parameter in a new instance, rather than being given a default of 4...
e.g. let someCar = Car(withDoors: X, andWheels: X, andSeats: X)
How would we go about writing the syntax for the initializer?
Apologies if it is really obvious! Just can't get my head round it!!!
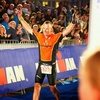
Steve Hunter
57,712 PointsHi Dominic,
I've not touched Swift in ages ... let me do some complete guesswork while I get my unused Xcode working!
I think you should pass the value you want for numberOfSeats
in with the initializer. Something like this - although I'm pretty sure this doesn't need the override
keyword as it isn't an override? I'll test it all in a bit when the updates have destroyed my bandwidth! ...
override init(numberOfSeats: Int, withDoors doors: Int, andWheels wheels: Int){
self.numberOfSeats = numberOfSeats
super.init(withDoors: doors, andWheels: wheels)
}
There's probably lots wrong with that (override and parameter names/labels for starters) ... I shall post again when I've got back into Swift mode and got Xcode running.
Steve.
Gregory Adams
7,416 PointsGregory Adams
7,416 PointsHi Steve,
I had nearly the same code as you, but the super.init was above the self.numberOfSeats attribution. I received the following 'error: property 'self.numberOfSeats' not initialized at super.init call'
Do you know why the order in the overriding init call matters?