Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial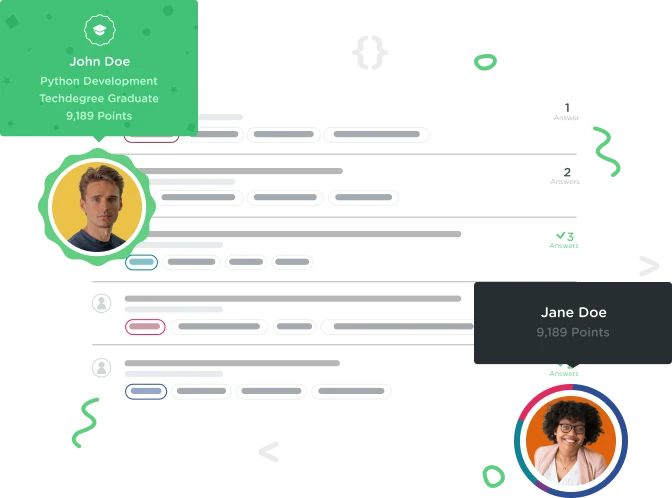

Ary de Oliveira
28,298 PointsSwift 2.0 Object Oriented
Challenge Task 2 of 2
Let's use the struct to create an instance of Person and assign it to a constant named aPerson. Assign any values you want to the first and last name properties.
Once you have an instance, call the instance method and assign the full name to a constant named fullName.
I miss same thing here.... confuso
struct Person {
let firstName: String
let lastName: String
func getFullName() -> String {
return "\(firstName) \(lastName)"
}
}
7 Answers
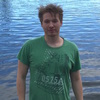
Emil Rais
26,873 PointsSupplied with the following struct:
struct Person {
let firstName: String
let lastName: String
func getFullName() -> String {
return "\(firstName) \(lastName)"
}
}
You should create a person and store it in a constant aPerson:
let aPerson = Person(firstName: "Ary", lastName: "de Oliveira")
You should then call its instance method (getFullName) and store it in a constant named fullName:
let fullName = aPerson.getFullName()
The fullName constant would now contain the value:
Ary de Oliveira
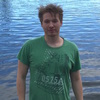
Emil Rais
26,873 PointsOkay, so you have a definition of a Person-struct. In Swift structs automatically have a constructor generated for them if one is not specified. The automatic constructor requires all fields specified upon creation. This particular struct has two fields, that need to be given a value upon creation: firstName and lastName.
The hidden constructor would look something like this:
init (firstName: String, lastName: String) {
self.firstName = firstName
self.lastName = lastName
}

Ary de Oliveira
28,298 PointsHi Emil thank you for reading my code, i tried your alternative but not yet completed the result.
Thank you for help me...

Ary de Oliveira
28,298 PointsCool...
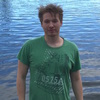
Emil Rais
26,873 PointsDid you figure it out?

Ary de Oliveira
28,298 PointsI am following you and trying to understand, thanks for the help, that complicated

Ary de Oliveira
28,298 PointsMy difficulty was to understand the context, thanks for your help. Pass Emil Cool....

Ary de Oliveira
28,298 PointsMr Emil Rais, once again thank you for your help, very cool...we have solved this team work
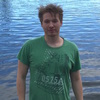
Emil Rais
26,873 PointsThat's great to hear. Happy learning.
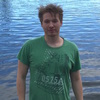
Emil Rais
26,873 PointsYou shouldn't write that particular constructor it is already there behind the scenes. What you need to do is create a person using the Person-struct and then get its full name and store it in some variable.
I'll show you how it is done. If you need more help don't be afraid to ask. You create structs using their constructors - the ones marked with the init keyword.
struct Dog {
let name: String
}
This Dog-struct has an implicit constructor (a hidden one that you can use) that requires a name. It looks something like this:
init (name: String) {
self.name = name
}
If I want to create a dog named Spot I would do it like this:
Dog(name: "Spot")

Ary de Oliveira
28,298 Pointsreflecting about your code ....