Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial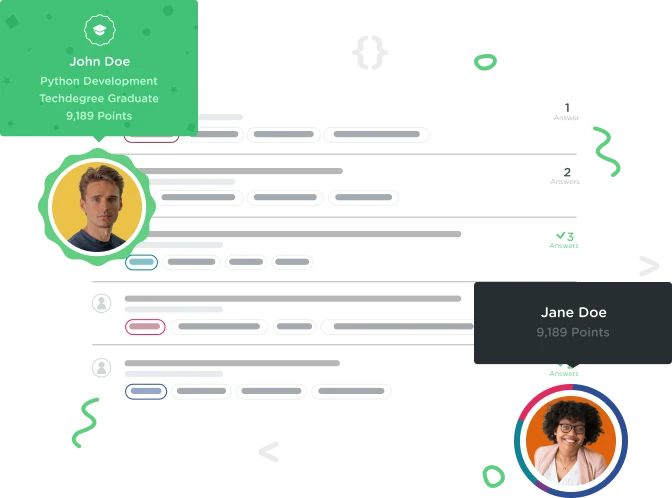
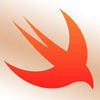
Jeff McDivitt
23,970 PointsSwift 3 generics 2 of 5
Can someone assist; as I believe I have the information correct but cannot pass
struct Queue<Element> {
var array: [Element]
var isEmpty: Bool {
return array.isEmpty
}
}
4 Answers
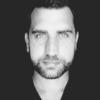
Jhoan Arango
14,575 PointsHello guys:
Going through your codes and I see you guys did great ??.
Now I would like to show you a different approach, and one that takes advantages of methods that are already in place.
struct Queue <Element> {
// Array
var array = [Element]()
// Computed Properties
var isEmpty: Bool {
return array.isEmpty
}
var count: Int {
return array.count
}
// Instance Methods
mutating func enqueue(_ element: Element) {
array.append(element)
}
mutating func dequeue() -> Element? {
return array.isEmpty ? nil : array.removeFirst()
}
}
As you may have noticed we are using the "isEmpty" bool to know if the array is empty or not, take advantage of this. Also noticed on the dequeue() method, that I'm using return array.isEmpty ? nil : array.removeFirst(). This is short for the if statement.
It basically says: if array.isEmpty, then return nil, otherwise remove the first element.
Hope this helps you look into cleaner and shorter code. With time you will get the hang of it.
Good luck on your challenges.
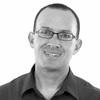
David Papandrew
8,386 PointsJeff,
This worked for me:
struct Queue<Element> {
//Task 1
var array: [Element] = []
//Task 2
var isEmpty: Bool {
if array.count == 0 {
return true
} else {
return false
}
}
}
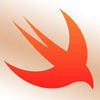
Jeff McDivitt
23,970 PointsDavid - Thank you very much. Could you assist me on the last part of the challenge. I got through step 3 and 4 but cannot seem to get Step 5 to work
struct Queue<Element> {
var array: [Element] = []
var isEmpty: Bool {
if array.count == 0 {
return true
} else {
return false
}
}
var count: Int {
return array.count
}
public mutating func enqueue(_ element: Element){
array.append(element)
}
public mutating func dequeue() -> Element? {
guard array.isEmpty, let element = array.first else { return nil }
array.remove(element)
return element
}
}
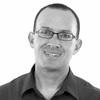
David Papandrew
8,386 PointsHi Jeff,
Hope this helps:
mutating func dequeue() -> Element? {
var firstItem: Element? = nil
if array[0] != nil {
firstItem = array[0]
self.array.removeFirst()
}
return firstItem
}