Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial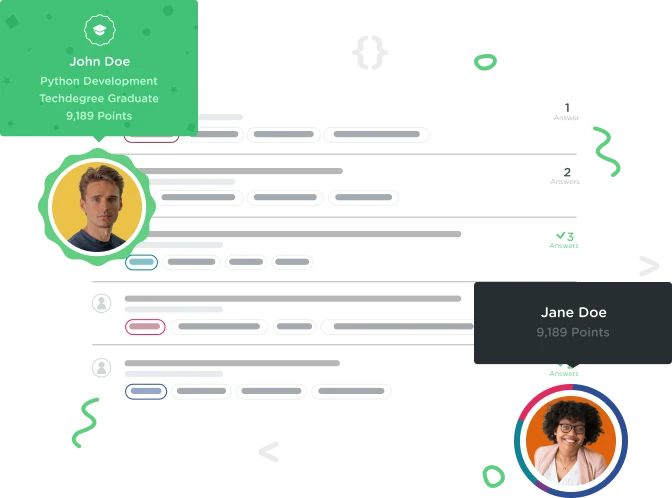

james gamber
7,727 Pointsswift 3 generics task
In the editor, define a function, named largest, with a generic type parameter, T. The function takes an array of type T as its first argument. Give this argument an external name of in. The return type of the function is optional T.
Given an array the function should return the largest value in the array. For example, calling largest(in: [1,2,3]) should return 3. To make this work, you'll need to constrain the generic type T to conform to Comparable.
func largest<T: Comparable>(in: [T]) -> T? {
var larger: T = 0 as! T
for var i in 0...`in`.count-1 {
if `in`[i] > larger {
larger = `in`[i]
i += 1
}
}
return larger
}
works in playground, doesn't work in challenge. Challenge give no details, error is "Bummer"
func largest<T: Comparable>(in: [T]) -> T? {
var larger: T = 0 as! T
for var i in 0...`in`.count-1 {
if `in`[i] > larger {
larger = `in`[i]
i += 1
}
}
return larger
}
largest(in: [1,2,3])
Jonathan Sweeney
14,559 PointsJonathan Sweeney
14,559 PointsHey realize this is late. I think I found a couple issues as to why your solution didn't pass the coding challenge.
Below is my solution. Hope this helps someone down the road!