Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial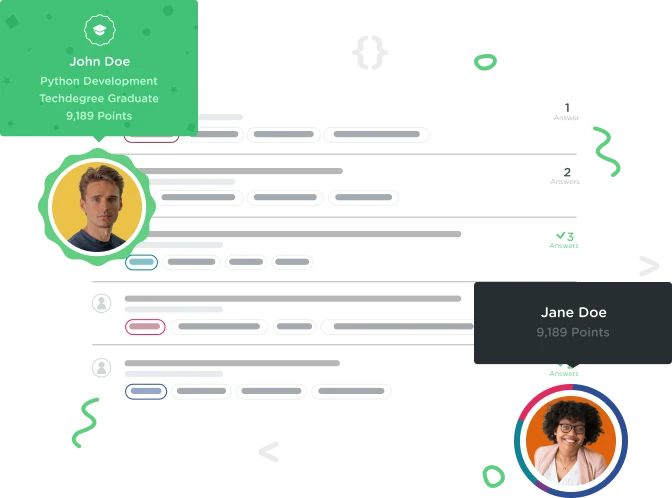
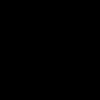
Michael Boswell
2,363 PointsSwift 3 Local Variable Parameter
Similarly to increment and decrement, Xcode is telling me of more changes coming in Swift 3.
In this video, Pasan says that an argument by default is a constant, and to change that you put var in front of it. He explains it as thus:
Constant
func countDownAndUp(a: Int) {
// do something
}
Variable
func countDownAndUp(var a: Int) {
// do something
}
With Swift 3 deprecating var here, does that mean it's a var by default?
1 Answer
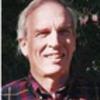
jcorum
71,830 PointsMichael, here's an excerpt from Swift.org (https://swift.org/blog/swift-2-2-new-features/):
var parameters are deprecated
Prior to Swift 2.2, function parameters could be declared as var if you wanted to modify them inside the function. For example:
func greet(var name: String) {
name = name.uppercaseString
print("Hello, \(name)!")
}
var name = "Taylor"
greet(name)
print("After function, name is \(name)")
While this was a helpful shortcut, it did add some extra confusion: does that final print() statement output “Taylor” or “TAYLOR”? This was made even more confusing by the presence of the inout keyword: using inout rather than var in that example, then adding a single ampersand, produces this code:
func greet(inout name: String) {
name = name.uppercaseString
print("Hello, \(name)!")
}
var name = "Taylor"
greet(&name)
print("After function, name is \(name)")
When run, the var example produces different output to the inout example, because changes to var parameters apply only inside the function whereas changes to inout parameters affect the original value directly.
In Swift 2.2, this confusion is cleared up by deprecating the var keyword for function parameters, ahead of its removal in Swift 3.0. If you want to replicate the old behavior, simply create your own copy inside the function like this:
func greet(name: String) {
let uppercaseName = name.uppercaseString
print("Hello, \(uppercaseName)!")
}
Looks like parameters will be constants unless marked inout.