Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial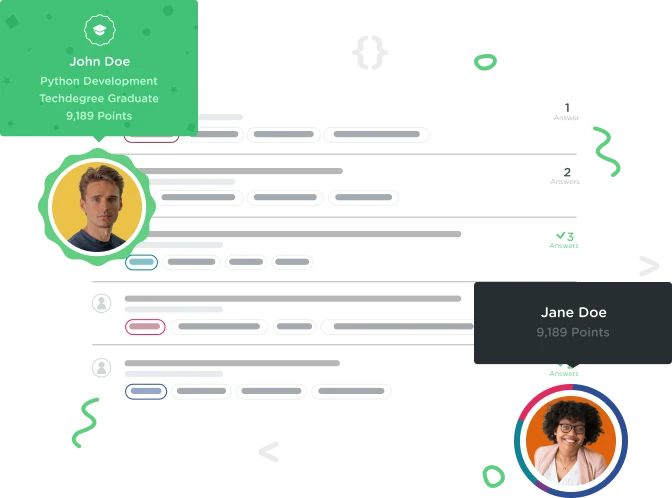

josh whitehead
479 Pointsswift challenge help
can somebody help me pass this challenge it is asking me to append the results of numbers between 1...100 if they are both odd and multiples of 7 and i have tried the .append method but i can't seem to get it right
var results: [Int] = [results.append(n)]
for n in 1...100 {
// Enter your code below
n % 2 != 0 && n % 7 == 0
}
5 Answers
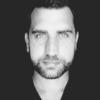
Jhoan Arango
14,575 PointsHello Josh,
You are close to completing your challenge. It seems as you may be lost a little in the appending part.
Remember, we are working with an array here and in order to append or add elements to an array you can use the "append" method that comes standard in arrays.
For example:
// An empty array
var someArray: [String] = []
// Adding an element to the array
someArray.append("Hello")
Now the array contains one element of type string.
So in your challenge every time the loop iterates through all the elements in the array ( in this case Ints from 1 to 100) we need to append the result.
var results: [Int] = []
for n in 1...100 {
// We check for the conditions to be true
if n % 2 != 0 && n % 7 == 0 {
// if true we append
results.append(n)
}
}
I hope this helps.

josh whitehead
479 Pointsyes thats jhoan i was using that exact code except I forgot to include the added {} to the results.append. Much appreciated thanks Josh
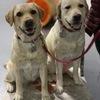
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Josh,
Let's step through your code line by line and maybe that will help to clarify where you're going wrong.
var results: [Int] = [results.append(n)]
The original code declared an empty array of type [Int]. Here you are declaring a variable called 'results', of type [Int] and with an initial value of [results.append(n)]. However, you can't pass to the variable you are creating a value that depends on results, because results doesn't exist yet. When doing an assignment, Swift, like most languages, computes the whole expression to the right of the assignment operator in order to get a value, and then takes that value and associates it with the name of your variable. But if variable results is being created from the result of computing the value to the right of the assignment operator, where is the results
on the right coming from? If this example seems too complex, consider the simple example and think about why this would give a 'variable used within its own initial value':
var my_variable = my_variable
The fundamental principle that you can't use a value that doesn't exist yet also applies to append(n)
part of the same line. Hopefully, if the previous issue makes sense to you, it should be obvious that you can't use n
before n
exists.
Let's move on to the next line:
for n in 1 ... 100 {
Here we're creating a for
loop. In Swift, variables declared in creating a loop only survive for the duration of that loop's iteration. And this is where we're bringing n
into existence. Your variable n
will only persist during the execution of the for loop.
Next line:
n % 2 != 0 && n % 7 == 0
This code is perfectly valid as far as it goes. However, let's think about what it is actually doing. First, it's executing n % 2 != 0
. The result of this will be True if n
is odd, False if it is even. You're also executing n % 7 == 0
. The result of this will be True if n
is evenly divisible by 7, and False otherwise. Finally, it's executing a boolean AND on those two values. So for values that are both odd and divisible by 7, it will resolve to True, otherwise False.
But that's it. Every time the compiler steps through your for loop, it's going to compute a True or False value, and then just move on to the next line. Just like if you wrote a line that was simply 'True' or a line that was simply 'False'. Swift would understand that but not do anything with it.
In your case you want to make a decision based on whether the Swift compiler saw a True or False, so you're going to need a conditional.
Now, let's imagine you had a conditional, and we're in the body of the conditional test (having resolved to True). At this point, we have a value n
(because we're in the for loop). And we know it meets our conditional test. So at this point, we probably want to put our value of n into our results variable. Fortunately, you already have the code for appending some value to an array, so you just need to put it here.
Hope this helps clear everything up. Let me know if you have any further questions
Cheers
Alex

josh whitehead
479 Pointshey alex
thanks a lot for your reply! I understand your breakdown of everything until the final part i still don't understand how to append the results of the loop to the results variable
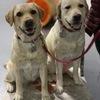
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Josh,
You do understand, as you've written exactly the right code! You've just put it in the wrong place.
Just to clarify, you're not appending the results of the loop, you're appending n
as it is during the loop. The loop itself has no value. n
has a value during each cycle of the loop.
So when you are in the loop you can use the append method on the results variable (remember that results was declared at the outermost level of the program so has global scope and is thus visible inside your loop) and the append method takes as its argument the value you want to append.

josh whitehead
479 PointsHey Alex, I am completely stuck on the final step and dont know what i am doing wrong. I keep getting the message: Make sure you're appending the correct values to the results array in order to pass the challenge Could you please illustrate how the whole code should look so i can get a visual understanding lol much appreciated Josh
josh whitehead
479 Pointsjosh whitehead
479 PointsHere is the challenge:
For this challenge, we'd like to know in a range of values from 1 to 100, how many numbers are both odd, and a multiple of 7.
To start us off, I've written a for loop to iterate over the desired range of values and named the local constant n. Your job is to write an if statement inside the for loop to carry out the desired checks.
If the number is both an odd number and a multiple of 7, append the value to the results array provided.
Hint: To check for an odd number use the not operator to check for "not even"