Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial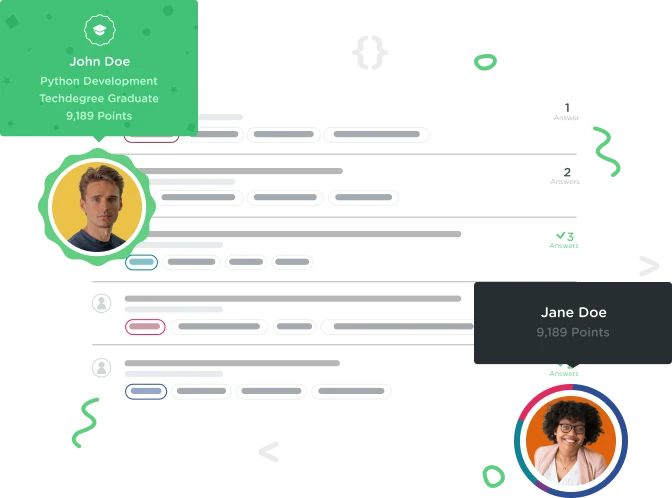

Ary de Oliveira
28,298 PointsSwift Challenges
I need help with these 2 challenges:
Challenge Task 1 of 2
This one might be a bit tricky so let's take it in steps. We have an array of numbers, nothing tricky, you've seen this before. What we want is to compute the sum of the numbers in the array. To do this we're going to use a while loop.
We have a variable ,sum, that will store the value of the sum of numbers from the array.
We also have a variable ,counter, which we will use to track the number of iterations of the while loop.
Step 1: Create a while loop. The while loop should continue as long as the value of counter is less than the number of items in the array. (Hint: You can get that number by using the count property)
my code no work
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Enter your code below
while counter < numbers.count {
print(numbers[counter])
counter++
}
sum =
Challenge Task 2 of 2
Now that we have the while loop set up, it's time to compute the sum! Using the value of counter as an index value, retrieve each value from the array and add it to the value of sum.
For example: sum = sum + newValue. Or you could use the compound addition operator sum += newValue where newValue is the value retrieved from the array.
no work BUMMMM
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Enter your code below
while counter < numbers.count { counter++}
while counter < numbers.count {
print(numbers[counter])
counter++
}
sum =
9 Answers
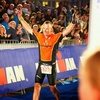
Steve Hunter
57,712 PointsI've put you a solution in your other post. Again, you're very nearly there - just slightly out on the while loop construction.
You've correctly identifed that you want to loop while counter
is less than numbers.count
. That's a great start. You then want to make sure you increment counter inside the loop else it'll never end!
Before that, take the array element and add it to sum
. The element is numbers[counter]
. That all comes out like:
while (counter < numbers.count){
sum += numbers[counter]
counter++
}
Steve.

Daniel Walker
2,031 Pointsthis way worked for me
Part 1/2
while counter < numbers.count
{ counter += 1}
Part 2/2
while counter < numbers.count
{ counter += 1
sum = sum + numbers[counter-1]
}

Ryan Rassoli
3,365 PointsCould you explain how you got line 2 on part 1?

Jordan John
iOS Development with Swift Techdegree Student 1,925 PointsThis worked great Daniel Walker . Thanks Much! I was really in a bind.

Blake Bassett
3,448 Pointswhile counter < numbers.count {
print(numbers[counter])
counter += 7
}
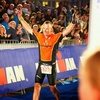
Steve Hunter
57,712 PointsHi Blake,
Why increment by 7?
Steve.
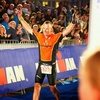
Steve Hunter
57,712 PointsHi Robert Hand ,
First of all, you're pretty much there with your code. But you don't want to print
anything. So, change your line:
print(sum += numbers[counter])
to:
sum += numbers[counter]
That should do it.
The reason you're unfamiliar with counter++
is because this was deprecated in Swift 3.0. The course that this thread was about was an earlier version of Swift where the unary increment operator, ++
, was allowed (as it is in most other languages). It was a convenient way of adding one to a number and came with its brother, --
, which did the opposite.
Let me know how you get on.
Steve.

Robert Hand
Courses Plus Student 2,657 PointsThat worked. Thanks so much for the quick response Steve!
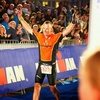
Steve Hunter
57,712 PointsNo problem!

michael duaybes
431 PointsWow thank you, I see how it is done now since counter is +1 in the array numbers. Nice!!!

Nathalie Dory
2,912 Pointsso while the variable -counter- (first at zero) is less that the total number of integers (there are 7) in the array -numbers, we are going to add the integer at position zero to sum and apply it back to the variable sum. The first integer at position zero is 2, so sum will now hold the value of two. We then add 1 to the counter and run the loop again, so while the counter (now valued at 1) is less than the total number of integers in the numbers array (still 7) we are going to add the sum, now valued at 2, to the integer at position one in the array, which is the second one, 8, which makes 10, which is applied back to sum, so the value of sum is now 10. Then we add one to the counter, making the counter 2 which is still less than 7 so we repeat until each integer is added to sum.
while counter < numbers.count { sum += numbers[counter]
counter+=1
}
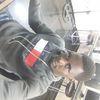
Kellington Chidza
866 Pointswhile counter < numbers.count {
print(numbers[counter])
counter += 1
}
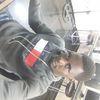
Kellington Chidza
866 Pointswhile counter < numbers.count { print(sum += numbers[counter]) counter += 1 }
Austin Lockard
2,936 PointsAustin Lockard
2,936 PointsThis may be a dumb question, but I need help understanding this statement: print(numbers[counter]). I get that numbers is a constant, so what does adding [counter] to the end of the statement actually do? Thank you
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsHi Austin Lockard ,
The constant,
numbers
is an array. It holds multiple values in a prescribed order. That order can be accessed by using a number to ask for the value stored at each particular place, i.e. 1st element, 2nd element etc. It has 7 elements in this example. Its definition is:let numbers = [2,8,1,16,4,3,9]
Each element of an array can be accessed by accessing its position within the array. We use the square brackets to do that, such that:
numbers[0]
holds the value 2.numbers[1]
holds the value 8.numbers[2]
holds the value 1.... and so on.
So, if we are looping, i.e. running the same portion of code repeatedly, and also incrementing the variable
counter
at each loop, usingcounter
to accessnumbers
allows us to iterate through every value thatnumbers
holds. Thecounter
is set to zero initially:counter
is 0 sonumbers[counter]
holds the value 2.counter
is 1 sonumbers[counter]
holds the value 8.counter
is 2 sonumbers[counter]
holds the value 1.What we then do with that value is irrelevant to the point. You can use
print
to display it on the screen or add it tosum
using+=
to add up the contents of the array. But that's what the square brackets, combined withcounter
, do.I hope that helps.
Steve.
Austin Lockard
2,936 PointsAustin Lockard
2,936 PointsThank you. That helps a ton. I just wasn't understanding where counter fit in the scheme of things. But I understand the logic behind it now. Thank you again.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsNo problem, Austin
Glad to help.
Steve.
Robert Hand
Courses Plus Student 2,657 PointsRobert Hand
Courses Plus Student 2,657 PointsI'm having trouble with Challenge Task 2 of 2...here's what I had for Task 1 of 2:
Task 2 - this is what I've tried and doesn't work:
Steve, I've tried the way as you've written it out and it's not working for me....I'm also not familiar with counter++